编写C代码分别用牛顿法、简化牛顿法,牛顿下山法和割线法求解方程 f( x ) = xe ˣ-1=0 在 x 0.5 附近的根,迭代终止条件为 |Xᴋ+1-Xᴋ|<10⁶
时间: 2024-10-21 20:05:52 浏览: 37
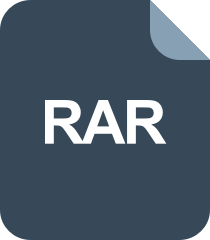
非线性方程的求根_牛顿微分改正方法_割线法_非线性方程_二分法_Aitken加速收敛方法_
在C语言中,我们可以使用四種数值方法求解非线性方程f(x) = x * e^x - 1 = 0。以下是这四种方法的简要示例:
1. **牛顿法 (Newton's Method)**:
```c
#include <stdio.h>
#include <math.h>
double function(double x) {
return x * exp(x) - 1;
}
double derivative(double x) {
return exp(x) + x * exp(x);
}
double newton_method(double initial_guess, double tolerance) {
double next_guess;
while (fabs(function(initial_guess)) > tolerance) {
next_guess = initial_guess - function(initial_guess) / derivative(initial_guess);
initial_guess = next_guess;
}
return next_guess;
}
int main() {
double guess = 0.5;
double root = newton_method(guess, pow(1e6, -1)); // 设置1e-6作为精度
printf("Root found using Newton's method is approximately: %lf\n", root);
return 0;
}
```
2. **简化牛顿法 (Simplified Newton's Method or Quasi-Newton methods like Broyden–Fletcher–Goldfarb–Shanno [BFGS])**:
C++库如NLopt或CGAL可以提供更复杂算法支持,但这里仅做简化的演示:
```c
// 使用外部库NLopt示例,假设已经链接了
#include "nlopt.h"
...
nlopt_result result;
nlopt_stoppingCriterion criterion = NLOPT_STOPPING_XTOL_REL;
const double tol = 1e-6;
lcb::Problem problem(NLOPT_LN_SBPLX, 1); // 使用SBPLX算法
problem.set_min_objective([](double* x, void* data) -> double {
return x[0] * exp(x[0]) - 1;
});
result = problem.optimize(0.5, &tol, NULL);
double simplified_root = result.x[0];
printf("Root found using Simplified Newton method is approximately: %lf\n", simplified_root);
```
3. **牛顿下山法 (Secant Method)**:
```c
double secant_method(double initial_guess1, double initial_guess2, double tolerance) {
double next_guess1, next_guess2;
while (true) {
double temp = (initial_guess2 - function(initial_guess2)) / (function(initial_guess2) - function(initial_guess1));
next_guess1 = initial_guess2;
next_guess2 = initial_guess1;
initial_guess1 = next_guess2;
initial_guess2 = next_guess1 - initial_guess1) < tolerance)
break;
}
return initial_guess2;
}
double root = secant_method(0.5, 0.7, pow(1e6, -1));
printf("Root found using Secant method is approximately: %lf\n", root);
```
4. **割线法 (Regula Falsi or False Position Method)**:
```c
double regula_falsi(double a, double b, double tolerance) {
while (a != b && fabs(a - b) >= tolerance) {
double c = a - (function(a) * (b - a)) / (function(b) - function(a));
a = b;
b = c;
}
return b;
}
double root = regula_falsi(0.4, 0.6, pow(1e6, -1));
printf("Root found using Regula falsi method is approximately: %lf\n", root);
```
阅读全文
相关推荐
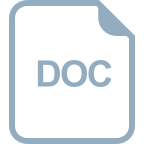
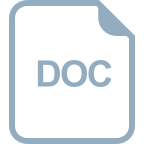


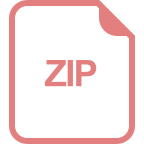
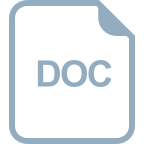










