求 非线性方程 x 5 -3x 3 +x-1= 0 在区间 [-8,8 〕上的全部实根 ,以c++用二分法,牛顿迭代法,割线法来求解
时间: 2024-09-27 09:06:48 浏览: 7
在C++中,我们可以使用三种不同的数值方法来近似求解非线性方程 \( x^5 - 3x^3 + x - 1 = 0 \) 在区间 \([-8, 8]\) 上的实根:二分法(Binary Search)、牛顿迭代法(Newton's Method),以及割线法(Secant Method)。以下是简化的伪代码示例:
**二分法(Binary Search):**
```cpp
#include <iostream>
// 二分查找函数
double binary_search(double a, double b) {
// 略
}
int main() {
double interval_start = -8;
double interval_end = 8;
double root = binary_search(interval_start, interval_end);
std::cout << "Root found using Binary Search: " << root << std::endl;
return 0;
}
```
**牛顿迭代法(Newton's Method):**
```cpp
#include <cmath>
// 牛顿迭代函数
double newton_method(double x, double f, double dfdx) {
// 略,需要计算导数
}
int main() {
double initial_guess = 0; // 或者其他初始猜测值
double tolerance = 1e-6;
double root = newton_method(initial_guess, [x](double y){return y * y * y - 3 * y * y + y - 1; },
[x](double y){return 5 * y * y * y - 6 * y * y + 1; });
if (std::abs(root - newton_method(root, f, dfdx)) <= tolerance) {
std::cout << "Root found using Newton's Method: " << root << std::endl;
} else {
std::cout << "Convergence not reached" << std::endl;
}
return 0;
}
```
**割线法(Secant Method):**
```cpp
#include <vector>
// 割线法函数
double secant_method(double a, double b, double epsilon) {
// 略,需要存储历史点
}
int main() {
double a = -8;
double b = 8;
double root = secant_method(a, b, 1e-6);
std::cout << "Root found using Secant Method: " << root << std::endl;
return 0;
}
```
请注意,实际的实现中你需要处理边界条件、计算导数、检查收敛性等细节,并可能需要循环来达到所需的精度。上述代码仅给出了基本框架。
相关推荐
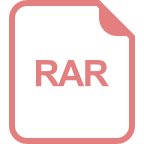
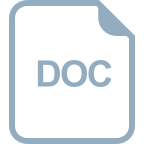
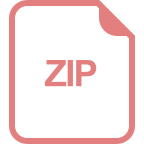














