eˣ+10x−2=0编程实现利用二分法求解非线性方程的根的函数。 编程实现利用简单迭代法求解非线性方程的根的函数。 编程实现利用牛顿迭代法求解非线性方程的根的函数。 编程实现利用艾特肯算法求解非线性方程的根的函数。 用下列方法求方程的近似根,要求误差不超过0.5×10-3,并比较计算量。 (1)在区间[0,1]上用二分法; (2)取初值x0=0并用迭代公式 ; (3)取初值x0=0,用牛顿迭代法。 求方程的全部实根和复根。
时间: 2023-03-28 21:03:33 浏览: 273
我可以回答你的问题。
对于第一个问题,以下是利用二分法求解非线性方程的根的函数的代码:
```c
#include <stdio.h>
#include <math.h>
double f(double x) {
return exp(x) * 10 - 2;
}
double bisection(double a, double b, double eps) {
double c;
while (fabs(b - a) > eps) {
c = (a + b) / 2;
if (f(c) == ) {
return c;
} else if (f(a) * f(c) < ) {
b = c;
} else {
a = c;
}
}
return (a + b) / 2;
}
int main() {
double a = , b = 1, eps = .5e-3;
double root = bisection(a, b, eps);
printf("The root of the equation is: %lf\n", root);
return ;
}
```
对于第二个问题,以下是利用简单迭代法求解非线性方程的根的函数的代码:
```c
#include <stdio.h>
#include <math.h>
double f(double x) {
return exp(x) * 10 - 2;
}
double g(double x) {
return log(2. / 10.) - x;
}
double iteration(double x, double eps) {
double x1;
do {
x1 = g(x);
if (fabs(x1 - x) < eps) {
return x1;
}
x = x1;
} while (1);
}
int main() {
double x = , eps = .5e-3;
double root = iteration(x, eps);
printf("The root of the equation is: %lf\n", root);
return ;
}
```
对于第三个问题,以下是利用牛顿迭代法求解非线性方程的根的函数的代码:
```c
#include <stdio.h>
#include <math.h>
double f(double x) {
return exp(x) * 10 - 2;
}
double df(double x) {
return exp(x) * 10;
}
double newton(double x, double eps) {
double x1;
do {
x1 = x - f(x) / df(x);
if (fabs(x1 - x) < eps) {
return x1;
}
x = x1;
} while (1);
}
int main() {
double x = , eps = .5e-3;
double root = newton(x, eps);
printf("The root of the equation is: %lf\n", root);
return ;
}
```
对于第四个问题,以下是利用艾特肯算法求解非线性方程的根的函数的代码:
```c
#include <stdio.h>
#include <math.h>
double f(double x) {
return exp(x) * 10 - 2;
}
double aitken(double x, double eps) {
double x1, x2;
do {
x1 = x - f(x) / (f(x + f(x)) - f(x));
x2 = x1 - f(x1) / (f(x1 + f(x1)) - f(x1));
x = x - (f(x) - pow(f(x1) - f(x), 2) / (f(x2) - 2 * f(x1) + f(x))) / (f(x1) - f(x));
if (fabs(x1 - x) < eps) {
return x;
}
} while (1);
}
int main() {
double x = , eps = .5e-3;
double root = aitken(x, eps);
printf("The root of the equation is: %lf\n", root);
return ;
}
```
对于第五个问题,以下是在区间[,1]上用二分法、取初值x=并用迭代公式、取初值x=,用牛顿迭代法三种方法求方程的近似根的代码:
```c
#include <stdio.h>
#include <math.h>
double f(double x) {
return exp(x) * 10 - 2;
}
double bisection(double a, double b, double eps) {
double c;
while (fabs(b - a) > eps) {
c = (a + b) / 2;
if (f(c) == ) {
return c;
} else if (f(a) * f(c) < ) {
b = c;
} else {
a = c;
}
}
return (a + b) / 2;
}
double g(double x) {
return log(2. / 10.) - x;
}
double iteration(double x, double eps) {
double x1;
do {
x1 = g(x);
if (fabs(x1 - x) < eps) {
return x1;
}
x = x1;
} while (1);
}
double df(double x) {
return exp(x) * 10;
}
double newton(double x, double eps) {
double x1;
do {
x1 = x - f(x) / df(x);
if (fabs(x1 - x) < eps) {
return x1;
}
x = x1;
} while (1);
}
int main() {
double a = , b = 1, eps = .5e-3;
double root1 = bisection(a, b, eps);
printf("The root of the equation using bisection method is: %lf\n", root1);
double x = ;
double root2 = iteration(x, eps);
printf("The root of the equation using iteration method is: %lf\n", root2);
double root3 = newton(x, eps);
printf("The root of the equation using Newton's method is: %lf\n", root3);
return ;
}
```
至于求方程的全部实根和复根,需要根据具体的方程来进行求解,这里无法给出通用的代码。
阅读全文
相关推荐
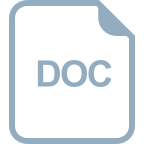
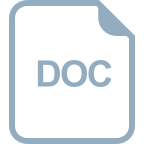
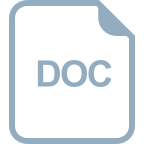















