写出后缀表达式求值的c++代码
时间: 2023-09-01 14:10:29 浏览: 132
好的,以下是一个简单的用栈实现的后缀表达式求值的C代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <ctype.h>
#define MAX_STACK_SIZE 100
typedef struct {
double data[MAX_STACK_SIZE];
int top;
} Stack;
void stack_init(Stack *s) {
s->top = -1;
}
int stack_is_full(const Stack *s) {
return s->top == MAX_STACK_SIZE - 1;
}
int stack_is_empty(const Stack *s) {
return s->top == -1;
}
double stack_peek(const Stack *s) {
if (stack_is_empty(s)) {
fprintf(stderr, "Stack is empty.\n");
exit(EXIT_FAILURE);
}
return s->data[s->top];
}
void stack_push(Stack *s, double value) {
if (stack_is_full(s)) {
fprintf(stderr, "Stack overflow.\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = value;
}
double stack_pop(Stack *s) {
if (stack_is_empty(s)) {
fprintf(stderr, "Stack is empty.\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
double evaluate_postfix(char *postfix) {
Stack s;
stack_init(&s);
while (*postfix) {
if (isdigit(*postfix)) {
double value = atof(postfix);
while (isdigit(*postfix) || *postfix == '.') postfix++;
stack_push(&s, value);
}
else if (*postfix == '+' || *postfix == '-' || *postfix == '*' || *postfix == '/') {
double b = stack_pop(&s);
double a = stack_pop(&s);
double result;
switch (*postfix) {
case '+': result = a + b; break;
case '-': result = a - b; break;
case '*': result = a * b; break;
case '/': result = a / b; break;
}
stack_push(&s, result);
postfix++;
}
else if (*postfix == ' ') {
postfix++;
}
else {
fprintf(stderr, "Invalid character: %c\n", *postfix);
exit(EXIT_FAILURE);
}
}
if (stack_is_empty(&s)) {
fprintf(stderr, "No result.\n");
exit(EXIT_FAILURE);
}
double result = stack_pop(&s);
if (!stack_is_empty(&s)) {
fprintf(stderr, "Too many values.\n");
exit(EXIT_FAILURE);
}
return result;
}
int main() {
char postfix[] = "3 2 + 4 *";
double result = evaluate_postfix(postfix);
printf("%f\n", result);
return 0;
}
```
这个代码先定义了一个栈结构体,包括一个数组和一个指向栈顶的索引;然后实现了基本的栈操作,包括初始化、判断栈是否满、判断栈是否空、返回栈顶元素、入栈、出栈等等。
evaluate_postfix 函数接收一个后缀表达式字符串作为参数,然后遍历这个字符串,分别处理数字和运算符。
当遇到数字时,先转换成 double 类型,然后入栈。
当遇到运算符时,先从栈中弹出操作数 b 和 a,然后根据运算符计算结果 result,最后把结果入栈。
当遇到空格时,直接跳过。
当遇到其他字符时,报错退出。
最后,返回栈顶元素作为结果。
阅读全文
相关推荐





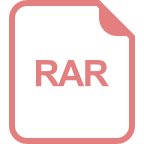












