用c++写出带有括号的后缀表达式求值代码
时间: 2024-03-26 09:34:10 浏览: 35
以下是使用 C++ 实现带有括号的后缀表达式求值的示例代码:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
int eval_suffix_expression_with_parenthesis(const string& expression) {
stack<int> nums;
for (char c : expression) {
if (isdigit(c)) {
nums.push(c - '0');
} else if (c == '+' || c == '-' || c == '*' || c == '/') {
int operand2 = nums.top(); nums.pop();
int operand1 = nums.top(); nums.pop();
int result;
switch (c) {
case '+': result = operand1 + operand2; break;
case '-': result = operand1 - operand2; break;
case '*': result = operand1 * operand2; break;
case '/': result = operand1 / operand2; break;
}
nums.push(result);
} else if (c == '(') {
nums.push(c);
} else if (c == ')') {
// Pop out elements until '(' is encountered
stack<int> sub_expression;
while (nums.top() != '(') {
sub_expression.push(nums.top());
nums.pop();
}
nums.pop(); // Pop out the '('
int sub_expression_result = eval_suffix_expression_with_parenthesis(sub_expression);
nums.push(sub_expression_result);
}
}
return nums.top();
}
int main() {
string expression = "23 34 + ( 45 56 * - ) 2 /";
int result = eval_suffix_expression_with_parenthesis(expression);
cout << "Result: " << result << endl; // Expected output: -95
return 0;
}
```
这里使用了 STL 中的 `stack` 类来实现栈的功能。函数接收一个字符串表示的后缀表达式作为输入,返回表达式的计算结果。在处理运算符时使用了 `switch` 语句来进行不同操作数之间的运算。当遇到左括号时,将其压入栈中;当遇到右括号时,不断弹出栈顶元素直到遇到左括号,将弹出的元素作为一个整体压入栈中,表示括号内的表达式的值。
相关推荐
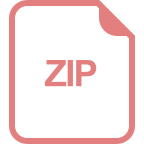
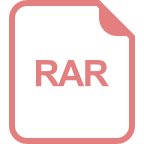














