用python game写扫雷游戏
时间: 2023-12-16 13:03:20 浏览: 108
非常好的问题!如果你想用 Python 编写扫雷游戏,可以考虑使用 Pygame 库。Pygame 是一个流行的 Python 游戏开发库,提供了许多有用的功能。你可以使用 Pygame 创建游戏窗口、绘制游戏元素、检测用户输入等。对于扫雷游戏,你可以使用 Pygame 实现游戏面板及其元素、计时器、背景音乐等,以提供更好的用户体验。祝你好运!
相关问题
用python写个扫雷游戏
可以的,我可以为您提供一个简单的代码示例,如下所示:
```
import random
def create_board(rows, cols, num_mines):
# Create an empty board
board = [[0 for col in range(cols)] for row in range(rows)]
# Add mines to the board
mines = 0
while mines < num_mines:
row = random.randint(0, rows - 1)
col = random.randint(0, cols - 1)
if board[row][col] != -1:
board[row][col] = -1
mines += 1
# Fill in the numbers around the mines
for row in range(rows):
for col in range(cols):
if board[row][col] == -1:
# Add 1 to each adjacent square
for i in range(-1, 2):
for j in range(-1, 2):
if 0 <= row + i < rows and 0 <= col + j < cols and board[row + i][col + j] != -1:
board[row + i][col + j] += 1
return board
def print_board(board):
rows = len(board)
cols = len(board[0])
# Print the column numbers
print(" ", end="")
for col in range(cols):
print(col, end=" ")
print("")
# Print the board
for row in range(rows):
print(row, end=" ")
for col in range(cols):
if board[row][col] == -1:
print("*", end=" ")
elif board[row][col] == 0:
print(".", end=" ")
else:
print(board[row][col], end=" ")
print("")
def reveal(board, revealed, row, col):
if revealed[row][col]:
return
rows = len(board)
cols = len(board[0])
# Reveal the square and continue recursively if it's a zero
revealed[row][col] = True
if board[row][col] == 0:
for i in range(-1, 2):
for j in range(-1, 2):
if 0 <= row + i < rows and 0 <= col + j < cols:
reveal(board, revealed, row + i, col + j)
def play_game():
rows = int(input("Enter the number of rows: "))
cols = int(input("Enter the number of columns: "))
num_mines = int(input("Enter the number of mines: "))
board = create_board(rows, cols, num_mines)
revealed = [[False for col in range(cols)] for row in range(rows)]
while True:
print_board(revealed)
row = int(input("Enter a row: "))
col = int(input("Enter a column: "))
if board[row][col] == -1:
print("You hit a mine! Game over.")
break
else:
reveal(board, revealed, row, col)
# Check if the game is won
if all(all(revealed[row][col] or board[row][col] == -1 for col in range(cols)) for row in range(rows)):
print_board(board)
print("You win!")
break
play_game()
```
该脚本将使用Python语言实现一个简单的扫雷游戏。用户将输入行数、列数和地雷数量,游戏板将被创建并显示。玩家可以选择一个单元格来揭开,如果点击地雷则游戏失败,如果玩家成功在未触雷的情况下揭示了所有方格,则获胜。
用python写扫雷游戏
好的,以下是一个简单的命令行扫雷游戏的实现,您可以参考一下:
```python
import random
def create_board(rows, cols, bombs):
# 初始化二维数组
board = [[0 for _ in range(cols)] for _ in range(rows)]
# 随机放置雷
locations = random.sample(range(rows*cols), bombs)
for loc in locations:
row = loc // cols
col = loc % cols
board[row][col] = "*"
# 对每个雷周围的格子数字加1
for r in range(row-1, row+2):
for c in range(col-1, col+2):
if r >= 0 and r < rows and c >= 0 and c < cols and board[r][c] != "*":
board[r][c] += 1
return board
def print_board(board):
for row in board:
print(" ".join(map(str, row)))
def play_game(rows, cols, bombs):
board = create_board(rows, cols, bombs)
revealed = [[False for _ in range(cols)] for _ in range(rows)]
game_over = False
while not game_over:
print_board(revealed)
row = int(input("Enter row: "))
col = int(input("Enter col: "))
if board[row][col] == "*":
print("Game over!")
game_over = True
else:
revealed[row][col] = True
if all(revealed[i][j] or board[i][j] != 0 for i in range(rows) for j in range(cols)):
print("You win!")
game_over = True
play_game(5, 5, 5)
```
这个实现中,我们通过 `create_board` 函数来随机生成扫雷游戏的地图,其中 `rows` 和 `cols` 分别是地图的行数和列数,`bombs` 是地图上的雷的数量。然后我们通过 `print_board` 函数来打印出当前已经翻开的格子,使用 `revealed` 数组来记录哪些格子已经翻开了。最后,我们通过 `play_game` 函数来实现游戏的主循环,让玩家输入坐标来翻开格子,同时判断游戏是否结束。
阅读全文
相关推荐
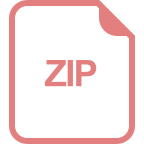


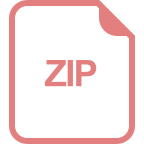










