Queue<TreeNode> queue=new LinkedList<>();为什么queue可以和list一起使用
时间: 2023-06-04 17:04:18 浏览: 117
这是因为LinkedList实现了Queue接口,所以可以通过将LinkedList对象赋给Queue类型的引用来使用。Queue接口提供了一些队列的基本操作方法,例如入队和出队。LinkedList实现了这些方法,因此可以通过LinkedList实例来创建一个Queue,这就是这个语句的作用。
相关问题
error: cannot find symbol queue<TreeNode> queue = new LinkedList<>();
This error occurs when the compiler cannot find the symbol or identifier used in the code. In this case, it is likely that the compiler cannot find the class or interface for either "queue" or "TreeNode".
To fix this error, make sure that the necessary libraries and dependencies are imported and that the class or interface is spelled correctly. In this case, it might be helpful to import the java.util.LinkedList and java.util.Queue libraries to ensure that the queue and TreeNode classes are recognized by the compiler. Additionally, make sure that the class or interface is defined and implemented correctly in the code.
Queue<TreeNode>
`Queue<TreeNode>`是一个队列,其中存储的元素类型是`TreeNode`。在Java中,`Queue`是一种接口,它定义了队列的基本操作,如入队、出队、查看队首元素等。`TreeNode`则是一个表示树节点的数据结构,通常用于树的遍历。
使用`Queue<TreeNode>`可以在树的遍历中实现广度优先搜索(BFS),即从根节点开始,先访问当前节点的所有子节点,再访问这些子节点的子节点,以此类推,直到遍历完整棵树。BFS通常使用队列来存储待访问的节点,每次从队列头部取出一个节点进行访问,并将其所有子节点加入队列尾部。
以下是一个简单的示例代码,使用`Queue<TreeNode>`实现树的BFS遍历:
```
public List<Integer> bfs(TreeNode root) {
List<Integer> result = new ArrayList<>();
Queue<TreeNode> queue = new LinkedList<>();
if (root != null) {
queue.offer(root); // 将根节点入队
}
while (!queue.isEmpty()) {
TreeNode node = queue.poll(); // 取出队首节点
result.add(node.val); // 访问当前节点
if (node.left != null) {
queue.offer(node.left); // 将左子节点入队
}
if (node.right != null) {
queue.offer(node.right); // 将右子节点入队
}
}
return result;
}
```
在上述代码中,`queue.offer()`方法用于将一个节点入队,`queue.poll()`方法用于取出队首节点并从队列中移除,`queue.isEmpty()`方法用于判断队列是否为空。这里的`TreeNode`表示树节点,包含一个整型值`val`和左右子节点的引用。`bfs()`方法接收一个根节点作为参数,返回一个整型列表,列表中存储了树的BFS遍历结果。
相关推荐
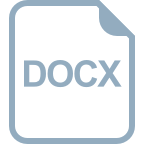
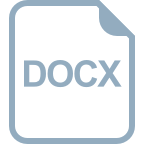
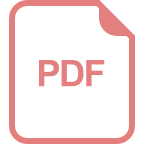












