C语言按序号按值查找查找
时间: 2024-09-30 11:09:49 浏览: 22
在C语言中,如果你需要按照特定的序号或值在一个数组或其他数据结构(如动态分配的内存块)中查找元素,你可以使用循环和条件语句(如`if`或`while`)来进行查找。以下是一个简单的示例,假设我们有一个整数数组:
```c
int numbers[] = {10, 20, 30, 40, 50};
int targetValue;
int index;
// 获取用户输入的目标值
scanf("%d", &targetValue);
// 按值查找
index = -1; // 初始化索引为 -1,表示未找到
for (size_t i = 0; i < sizeof(numbers) / sizeof(int); ++i) {
if (numbers[i] == targetValue) {
index = i; // 找到目标值,更新索引
break; // 一旦找到就退出循环
}
}
if (index != -1) {
printf("找到了,位置索引为 %d\n", index);
} else {
printf("没找到该值\n");
}
```
在这个例子中,程序会遍历数组,如果遇到等于`targetValue`的元素,就会停止搜索并返回其索引。如果没有找到,`index`将保持初始的 `-1` 值。
相关问题
c语言按序号查找字符顺序表代码
以下是一个简单的 C 语言按序号查找字符顺序表的代码示例:
```c
#include <stdio.h>
#define MAX_SIZE 100
typedef struct {
char data[MAX_SIZE];
int length;
} SeqList;
int locate(SeqList list, char target) {
for (int i = 0; i < list.length; i++) {
if (list.data[i] == target) {
return i;
}
}
return -1;
}
int main() {
SeqList list = {"Hello, world!", 13};
char target = 'o';
int index = locate(list, target);
if (index == -1) {
printf("The target character '%c' is not found.\n", target);
} else {
printf("The target character '%c' is found at index %d.\n", target, index);
}
return 0;
}
```
这个程序定义了一个 SeqList 结构体,其中包含一个字符数组和一个整数表示数组的长度。locate 函数接受一个 SeqList 和一个目标字符作为参数,返回目标字符在数组中的下标,如果找不到则返回 -1。在主函数中,我们创建了一个 SeqList 对象并调用 locate 函数来查找字符 'o' 在数组中的位置。
单链表按序号查找结点值c语言
实现代码如下:
```
#include <stdio.h>
#include <stdlib.h>
struct ListNode {
int val;
struct ListNode *next;
};
struct ListNode* createList(int arr[], int n) {
struct ListNode* head = NULL, *tail = NULL;
for (int i = 0; i < n; i++) {
struct ListNode* node = (struct ListNode*)malloc(sizeof(struct ListNode));
node->val = arr[i];
node->next = NULL;
if (head == NULL) {
head = tail = node;
} else {
tail->next = node;
tail = node;
}
}
return head;
}
int searchNode(struct ListNode* head, int pos) {
struct ListNode* p = head;
int cnt = 0;
while (p != NULL && cnt < pos) {
cnt++;
p = p->next;
}
if (p == NULL) {
return -1;
} else {
return p->val;
}
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int n = sizeof(arr) / sizeof(arr[0]);
struct ListNode* head = createList(arr, n);
int pos = 3;
int val = searchNode(head, pos);
if (val == -1) {
printf("不存在这个节点\n");
} else {
printf("第%d个节点的值为%d\n", pos, val);
}
return 0;
}
```
这是一个基于C语言的单链表结构实现,其中createList函数用于创建一个单链表,searchNode函数用于按序号查找链表中的节点值。
阅读全文
相关推荐
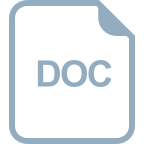
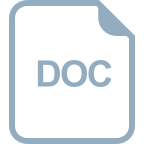
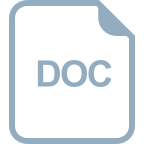













