输入线性表的各个数据域的值,同时显示创建的顺序表和链表结点数据;实现在第i个结点的之前插入一个新的结点,并显示插入后的线性表结点情况;实现将第i个结点删除,并将删除后的线性表结点情况显示出来;实现对线性表的按值查找和按序号查找。
时间: 2024-10-17 19:11:40 浏览: 30
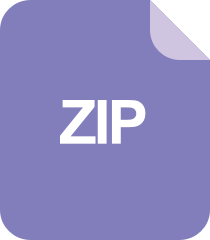
数据结构实验线性表(用java创建顺序表与链表并解决实验题目)

在输入线性表时,通常会包括每个结点的数据域(如整数、字符串等),以及其结构相关的属性。对于顺序表(数组形式),我们可以直接存储这些值并通过索引访问。例如:
```python
顺序表示例:
[5, 10, 15, 20]
```
而链表则包含节点结构,每个节点有值和指向下一个节点的指针:
```python
链表示例:
头结点 -> [1] -> [5] -> [10] -> [15] -> None
```
插入操作会在指定位置创建新节点,例如,在第i个节点前插入:
```python
def insert_at_index(lst, value, index):
new_node = Node(value)
if index == 0:
new_node.next = lst.head
lst.head = new_node
else:
current = lst.head
for _ in range(index - 1):
current = current.next
new_node.next = current.next
current.next = new_node
insert_at_index(linkedList, 8, 2) # 结果:[1, 8, 5, 10, 15, ...]
```
删除操作会移除指定位置的节点:
```python
def delete_at_index(lst, index):
if index == 0:
lst.head = lst.head.next
else:
current = lst.head
for _ in range(index - 1):
current = current.next
current.next = current.next.next
delete_at_index(linkedList, 2) # 结果:[1, 5, 10, 15, ...]
```
查找操作包括值查找和序号查找,比如:
```python
def search_by_value(lst, target):
for i, node in enumerate(lst):
if node.value == target:
return i
return -1 # 如果未找到,则返回-1
def search_by_index(lst, index):
if index < len(lst):
return lst[index].value
else:
raise IndexError("Index out of range")
```
阅读全文
相关推荐
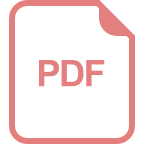

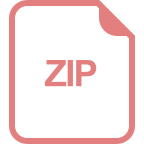
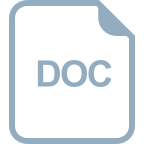
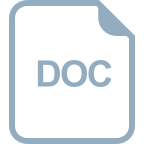
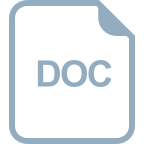
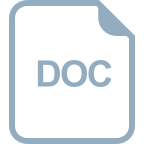
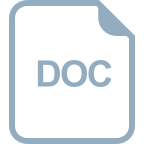
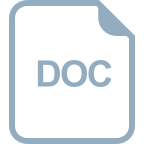
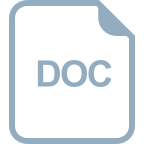
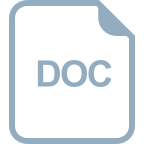
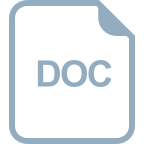
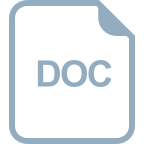
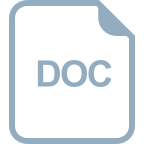


