实现三维变换的python代码
时间: 2024-10-19 20:12:56 浏览: 17
在Python中,可以使用`numpy`库来方便地处理三维变换。这里是一个简单的例子,展示如何创建一个变换矩阵并应用于三维向量:
```python
import numpy as np
# 创建基本变换矩阵
def create_transform_matrix(translate, rotate, scale):
# 平移
translation = np.identity(4)
translation[:3, 3] = translate # 假设translate是(x, y, z)
# 旋转
rotation_matrix = np.eye(4)
axis_angle = np.radians(rotate) # 将角度转换为弧度
axis, angle = axis_angle # 假设rotate是围绕z轴的一个角度
Rx = np.array([[1, 0, 0], [0, np.cos(angle), -np.sin(angle)], [0, np.sin(angle), np.cos(angle)]])
Rz = np.array([[np.cos(angle), -np.sin(angle), 0], [np.sin(angle), np.cos(angle), 0], [0, 0, 1]])
rotation_matrix[:3, :3] = np.dot(Rz, Rx)
# 缩放
scaling = np.identity(4)
scaling[:3, :3] *= scale # 假设scale是一个长度为3的列表,对应x、y、z的缩放比例
return np.dot(scaling, np.dot(rotation_matrix, translation))
# 应用变换
transformed_vector = create_transform_matrix((1, 2, 3), (45, 0), (1, 1, 1)).dot(np.array([1, 2, 3, 1])) # 1, 2, 3是对原点的初始位姿
```
在这个例子中,我们首先定义了三种变换的函数,然后通过矩阵乘法将它们组合在一起形成最终的变换矩阵。最后,这个矩阵用于乘以三维向量,得到变换后的结果。
阅读全文
相关推荐
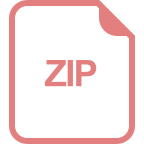
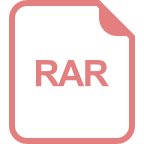
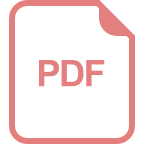















