怎么创建一个二维数组保存需要绘制多边形的顶点坐标
时间: 2024-03-21 15:38:43 浏览: 14
您可以使用以下代码创建一个二维数组来保存需要绘制多边形的顶点坐标:
```python
# 创建一个包含n个顶点的多边形,顶点坐标分别为(x1,y1), (x2,y2), ..., (xn,yn)
n = 4
vertices = [[x1, y1], [x2, y2], [x3, y3], [x4, y4]]
# 创建一个二维数组来保存顶点坐标
polygon = [[] for i in range(n)]
# 将顶点坐标添加到数组中
for i in range(n):
polygon[i] = vertices[i]
# 打印多边形的顶点坐标
print(polygon)
```
这样,您就可以使用 `polygon` 数组来保存多边形的顶点坐标,并对其进行绘制操作。
相关问题
np创建一个二维数组
使用NumPy可以通过多种方式创建二维数组。其中一些方法包括:
1. 使用np.array函数从列表或元组创建二维数组。例如,可以使用以下代码创建一个二维数组:
```
arr = np.array(\[\[1, 2, 3\], \[4, 5, 6\]\])
```
这将创建一个形状为(2, 3)的二维数组,其中包含两行三列的元素。
2. 使用np.zeros函数创建一个全零的二维数组。例如,可以使用以下代码创建一个形状为(3, 4)的全零二维数组:
```
arr = np.zeros((3, 4))
```
这将创建一个包含3行4列的二维数组,其中所有元素都为0。
3. 使用np.ones函数创建一个全1的二维数组。例如,可以使用以下代码创建一个形状为(3, 4)的全1二维数组:
```
arr = np.ones((3, 4))
```
这将创建一个包含3行4列的二维数组,其中所有元素都为1。
4. 使用np.random.rand函数创建一个随机的二维数组。例如,可以使用以下代码创建一个形状为(2, 3)的随机二维数组:
```
arr = np.random.rand(2, 3)
```
这将创建一个包含2行3列的二维数组,其中的元素是在0到1之间的随机数。
这些只是创建二维数组的一些方法,还有其他方法可以根据需要选择。\[1\]\[2\]\[3\]
#### 引用[.reference_title]
- *1* [numpy2](https://blog.csdn.net/qq_36264495/article/details/80275488)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
- *2* *3* [Python 创建二维数组](https://blog.csdn.net/flyingluohaipeng/article/details/129828525)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v91^koosearch_v1,239^v3^insert_chatgpt"}} ] [.reference_item]
[ .reference_list ]
如何创建一个二维数组?
创建一个二维数组可以使用以下步骤:
1. 首先,确定数组的行数和列数。
2. 使用数组类型声明一个二维数组变量,指定行数和列数。例如,int[][] array = new int[行数][列数]。
3. 使用嵌套的循环来为数组的每个元素赋值或访问元素。外层循环用于遍历行,内层循环用于遍历列。
4. 可以使用索引来访问特定位置的元素,例如array[行索引][列索引]。
下面是一个示例代码,演示了如何创建一个3行4列的二维整数数组并为其赋值:
```java
int[][] array = new int[3][4];
array[0][0] = 1;
array[0][1] = 2;
array[0][2] = 3;
array[0][3] = 4;
array[1][0] = 5;
array[1][1] = 6;
array[1][2] = 7;
array[1][3] = 8;
array[2][0] = 9;
array[2][1] = 10;
array[2][2] = 11;
array[2][3] = 12;
```
这样就创建了一个3行4列的二维数组,并为其赋值。你可以根据需要修改行数、列数和元素的值。
相关推荐
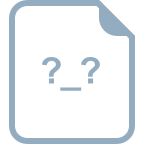
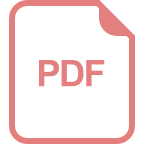












