在stm32103f中实现28byj-48步进电机控制的代码
时间: 2023-09-11 08:11:20 浏览: 166
以下是一个简单的代码示例,用于在STM32F103中控制28BYJ-48步进电机:
```
#include "stm32f10x.h"
#define STEPPER_PORT GPIOA
#define STEPPER_PIN_1 GPIO_Pin_0
#define STEPPER_PIN_2 GPIO_Pin_1
#define STEPPER_PIN_3 GPIO_Pin_2
#define STEPPER_PIN_4 GPIO_Pin_3
// 步进电机的状态
uint8_t stepper_states[4] = {0x01, 0x02, 0x04, 0x08};
uint8_t current_state = 0;
void stepper_init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
// 初始化GPIO
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
GPIO_InitStructure.GPIO_Pin = STEPPER_PIN_1 | STEPPER_PIN_2 | STEPPER_PIN_3 | STEPPER_PIN_4;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(STEPPER_PORT, &GPIO_InitStructure);
}
void stepper_step(uint8_t direction) {
// 计算下一个状态
if (direction == 0) {
current_state = current_state + 1;
} else {
current_state = current_state - 1;
}
// 如果超出状态数组范围,则重新从0开始
if (current_state > 3) {
current_state = 0;
} else if (current_state < 0) {
current_state = 3;
}
// 更新GPIO输出状态
GPIO_WriteBit(STEPPER_PORT, STEPPER_PIN_1, (stepper_states[current_state] & 0x01));
GPIO_WriteBit(STEPPER_PORT, STEPPER_PIN_2, (stepper_states[current_state] & 0x02));
GPIO_WriteBit(STEPPER_PORT, STEPPER_PIN_3, (stepper_states[current_state] & 0x04));
GPIO_WriteBit(STEPPER_PORT, STEPPER_PIN_4, (stepper_states[current_state] & 0x08));
}
int main(void) {
stepper_init();
// 步进电机顺时针旋转
for (int i = 0; i < 2048; i++) {
stepper_step(0);
for (int j = 0; j < 1000; j++);
}
// 步进电机逆时针旋转
for (int i = 0; i < 2048; i++) {
stepper_step(1);
for (int j = 0; j < 1000; j++);
}
while (1);
return 0;
}
```
这段代码可以让步进电机顺时针旋转2048步,然后逆时针旋转2048步。如果需要控制步进电机旋转的速度和精度,可以根据实际情况进行调整。
阅读全文
相关推荐
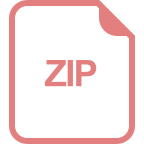




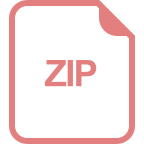
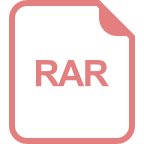
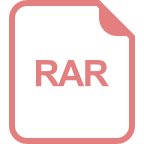
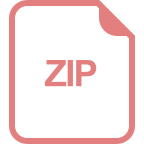
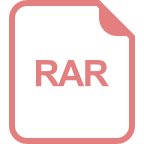
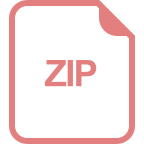
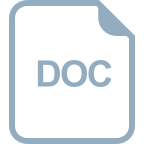
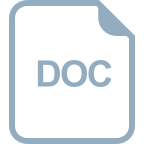



