for filename in images: img = cv2.imread(filename) temp_img = img.copy()
时间: 2024-05-18 12:14:57 浏览: 16
您好!您的第一个问题是关于Python代码的。这段代码使用OpenCV库读取一组图像,然后对每个图像进行副本处理。具体来说,它使用cv2.imread()函数读取图像,将其存储在img变量中,并使用.copy()方法创建一个名为temp_img的图像副本。这样做是为了在处理图像时不会修改原始图像。这段代码可以用于图像处理和计算机视觉应用程序中。希望能够帮助您!
相关问题
for i0, i in enumerate(img_set): img0 = cv2.imread(i)什么意思
这是一段 Python 代码,主要完成以下操作:
1. 遍历一个名为 `img_set` 的列表,其中每个元素都是一个图片的路径;
2. 对于遍历到的每个路径 `i`,使用 OpenCV 库的 `cv2.imread()` 函数读取对应的图片,并将其存储在变量 `img0` 中;
3. 在遍历过程中,使用 `enumerate()` 函数获取当前遍历到的元素的索引 `i0`。
因此,这段代码的主要作用是读取一组图片,并且记录每个图片在列表中的索引。
def imread(filename, flatten=False): """根据图片路径,将图片读取为cv2的图片处理格式.""" if not os.path.isfile(filename): raise FileNotExistError("File not exist: %s" % filename) # choose image readin mode: cv2.IMREAD_UNCHANGED=-1, cv2.IMREAD_GRAYSCALE=0, cv2.IMREAD_COLOR=1, readin_mode = cv2.IMREAD_GRAYSCALE if flatten else cv2.IMREAD_COLOR if PY3: img = cv2.imdecode(np.fromfile(filename, dtype=np.uint8), readin_mode) else: filename = filename.encode(sys.getfilesystemencoding()) img = cv2.imread(filename, readin_mode) return img
这是一个名为imread的函数,用于读取图像并将其转换为OpenCV的图像处理格式。函数接受两个参数:filename(图片路径)和flatten(是否将图像展平,默认为False)。
函数首先检查给定的文件是否存在,如果不存在,则会引发一个FileNotExistError异常。
然后,函数根据flatten参数的值选择图像读取模式。如果flatten为True,则选择cv2.IMREAD_GRAYSCALE,将图像解码为灰度图像;否则,选择cv2.IMREAD_COLOR,将图像解码为彩色图像。
接下来,函数使用cv2.imdecode函数将图像解码为OpenCV的图像格式。在Python 3中,使用np.fromfile函数从文件中读取图像数据,并使用cv2.imdecode进行解码。在Python 2中,首先将文件名编码为系统文件编码格式,然后使用cv2.imread函数进行解码。
最后,函数返回解码后的图像。
这个函数可以方便地读取图像并根据需要选择解码方式(灰度或彩色)。
相关推荐
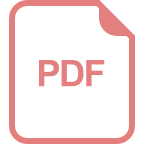
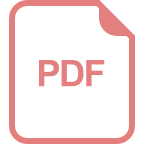
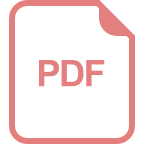
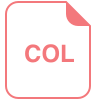
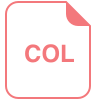
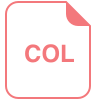
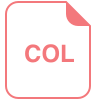
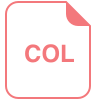







