python实现二次插值
时间: 2024-09-26 11:08:49 浏览: 5
在Python中,二次插值(Quadratic Interpolation)通常用于估算给定数据点之间的一个连续函数的近似值。当你只有三个已知的数据点,比如 (x1, y1), (x2, y2), 和 (x3, y3),并且这些点呈线性排列,那么可以使用二次多项式来拟合这个区域。最简单的方法是利用公式:
\[ f(x) = a * (x - x2)^2 + b * (x - x2) + c \]
其中 \(a\), \(b\), 和 \(c\) 可以通过下面的计算得出:
1. \( a = \frac{(y2 - y1)(x3 - x2) - (y3 - y1)(x2 - x1)}{(x2 - x1)^2 * (x3 - x2)} \)
2. \( b = \frac{-2 * a * (x1*(y2-y3)) + (y3 - y1)*(x2+x3-x1)}{(x2 - x1) * (x3 - x2)} \)
3. \( c = y1 - a * (x1 - x2)^2 - b * (x1 - x2) \)
你可以编写一个小函数来实现这个过程,示例如下:
```python
def quadratic_interpolation(x1, y1, x2, y2, x3, y3):
if x1 == x2 and x2 == x3: # 如果数据点完全相同,抛出异常
raise ValueError("Data points must be distinct.")
a = ((y2 - y1) * (x3 - x2) - (y3 - y1) * (x2 - x1)) / ((x2 - x1) ** 2 * (x3 - x2))
b = (-2 * a * (x1 * (y2 - y3)) + (y3 - y1) * (x2 + x3 - x1)) / ((x2 - x1) * (x3 - x2))
c = y1 - a * (x1 - x2)**2 - b * (x1 - x2)
return a, b, c
# 使用示例
x_values = [1, 2, 4]
y_values = [0, 1, 4]
x = 3 # 需要插值的点
a, b, c = quadratic_interpolation(*zip(x_values, y_values))
estimated_y = a * (x - x_values[1])**2 + b * (x - x_values[1]) + c
```
相关推荐
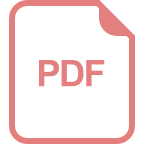
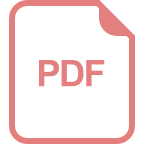
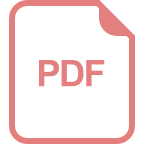
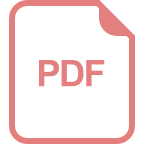
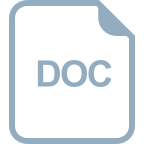
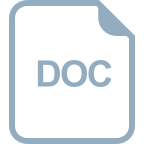











