输入无向图的顶点数、边数及各条边的顶点对,用c语言建立用邻接表表示的无向图
时间: 2024-02-15 13:05:29 浏览: 25
好的,以下是用C语言建立用邻接表表示的无向图的代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 边节点
typedef struct EdgeNode {
int adjvex; // 邻接点编号
struct EdgeNode *next; // 指向下一个边节点的指针
} EdgeNode;
// 顶点节点
typedef struct VertexNode {
int data; // 顶点数据(编号)
EdgeNode *firstedge; // 指向第一个边节点的指针
} VertexNode;
// 邻接表
typedef struct {
VertexNode vexs[100]; // 顶点数组
int vexnum; // 顶点数
int edgenum; // 边数
} Graph;
// 初始化邻接表
void InitGraph(Graph *G, int n) {
G->vexnum = n;
G->edgenum = 0;
for (int i = 0; i < n; i++) {
G->vexs[i].data = i;
G->vexs[i].firstedge = NULL;
}
}
// 插入边节点
void InsertEdge(Graph *G, int u, int v) {
// 创建新的边节点
EdgeNode *e = (EdgeNode*)malloc(sizeof(EdgeNode));
e->adjvex = v;
e->next = G->vexs[u].firstedge;
G->vexs[u].firstedge = e;
// 由于是无向图,需要插入反向边
e = (EdgeNode*)malloc(sizeof(EdgeNode));
e->adjvex = u;
e->next = G->vexs[v].firstedge;
G->vexs[v].firstedge = e;
G->edgenum++;
}
int main() {
int n, m; // n为顶点数,m为边数
int u, v;
printf("请输入顶点数和边数:");
scanf("%d %d", &n, &m);
Graph G;
InitGraph(&G, n);
printf("请输入每条边的顶点对:\n");
for (int i = 0; i < m; i++) {
scanf("%d %d", &u, &v);
InsertEdge(&G, u, v);
}
printf("邻接表如下:\n");
for (int i = 0; i < n; i++) {
printf("%d: ", i);
EdgeNode *e = G.vexs[i].firstedge;
while (e) {
printf("%d ", e->adjvex);
e = e->next;
}
printf("\n");
}
return 0;
}
```
这段代码可以根据用户输入的顶点数和边数,以及每条边的顶点对来建立用邻接表表示的无向图,并将邻接表输出。注意,这里假设顶点的编号从0开始。
相关推荐
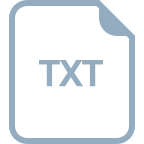
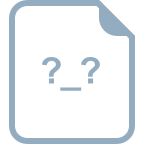













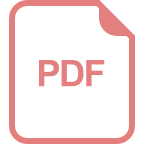