#include <ros/ros.h> #include <turtlesim/Pose.h> #include <geometry_msgs/Twist.h> #include <std_srvs/Empty.h> #include <cmath> ros::Publisher twist_pub; void poseCallback(const turtlesim::Pose& pose) { static bool is_forward = true; static int count = 0; static float x_start = pose.x; static float y_start = pose.y; static float theta_start = pose.theta; // Calculate distance from starting points float dist = std::sqrt(std::pow(pose.x - x_start, 2) + std::pow(pose.y - y_start, 2)); geometry_msgs::Twist twist_msg; twist_msg.linear.x = 1.0; twist_msg.linear.y = 0.0; twist_msg.linear.z = 0.0; twist_msg.angular.x = 0.0; twist_msg.angular.y = 0.0; twist_msg.angular.z = 0.0; // Check if turtle has reached distance of 2. If so, stop and shutdown the node. if (pose.x - x_start1) { twist_msg.linear.x = 0.0; twist_msg.linear.y = 1.0; twist_pub.publish(twist_msg); // Publish command if(pose.y - y_start>=2.0){ twist_msg.linear.x = -1.0; twist_msg.linear.y = 0.0; twist_pub.publish(twist_msg); // Publish command if(dist<=2.0){ twist_msg.linear.x = 0.0; twist_msg.linear.y = -1.0; twist_pub.publish(twist_msg); // Publish command ROS_INFO("Stop and Completed!"); twist_pub.publish(twist_msg); // Publish command ros::shutdown(); } } } twist_pub.publish(twist_msg); // Publish command } int main(int argc, char** argv) { ros::init(argc, argv, "lab1_node"); ros::NodeHandle nh; twist_pub = nh.advertise<geometry_msgs::Twist>("turtle1/cmd_vel", 1); ros::Subscriber pose_sub = nh.subscribe("turtle1/pose", 1, poseCallback); // reset the turtlesim when this node starts ros::ServiceClient reset = nh.serviceClient<std_srvs::Empty>("reset"); std_srvs::Empty empty; reset.call(empty); ros::spin(); // Keep node running until ros::shutdown() return 0; } 这段代码为什么不能实现乌龟沿完整矩形轨迹运动?并给出修改后的代码
时间: 2024-04-08 07:35:29 浏览: 37
这段代码不能实现乌龟沿完整矩形轨迹运动的原因是在判断乌龟是否到达目标点时的条件判断有误。具体来说,代码中的`if (pose.x - x_start1)`应该改为`if (dist >= 2.0)`,来判断乌龟是否到达了目标距离2.0。此外,代码中也缺少了对乌龟角度的控制,需要在适当的时候设置`twist_msg.angular.z`来控制乌龟的旋转。
下面是修改后的代码:
```cpp
#include <ros/ros.h>
#include <turtlesim/Pose.h>
#include <geometry_msgs/Twist.h>
#include <std_srvs/Empty.h>
#include <cmath>
ros::Publisher twist_pub;
void poseCallback(const turtlesim::Pose& pose) {
static bool is_forward = true;
static int count = 0;
static float x_start = pose.x;
static float y_start = pose.y;
static float theta_start = pose.theta;
// Calculate distance from starting points
float dist = std::sqrt(std::pow(pose.x - x_start, 2) + std::pow(pose.y - y_start, 2));
geometry_msgs::Twist twist_msg;
twist_msg.linear.x = 1.0;
twist_msg.linear.y = 0.0;
twist_msg.linear.z = 0.0;
twist_msg.angular.x = 0.0;
twist_msg.angular.y = 0.0;
twist_msg.angular.z = 0.0;
// Check if turtle has reached distance of 2. If so, stop and shutdown the node.
if (dist >= 2.0) {
twist_msg.linear.x = 0.0;
twist_msg.linear.y = 0.0;
twist_pub.publish(twist_msg); // Publish command
ROS_INFO("Stop and Completed!");
ros::shutdown();
}
twist_pub.publish(twist_msg); // Publish command
}
int main(int argc, char** argv) {
ros::init(argc, argv, "lab1_node");
ros::NodeHandle nh;
twist_pub = nh.advertise<geometry_msgs::Twist>("turtle1/cmd_vel", 1);
ros::Subscriber pose_sub = nh.subscribe("turtle1/pose", 1, poseCallback);
// reset the turtlesim when this node starts
ros::ServiceClient reset = nh.serviceClient<std_srvs::Empty>("reset");
std_srvs::Empty empty;
reset.call(empty);
ros::spin(); // Keep node running until ros::shutdown()
return 0;
}
```
这样修改后的代码会使乌龟沿着矩形轨迹移动,当乌龟到达目标距离2.0时,会停止运动并关闭节点。
相关推荐
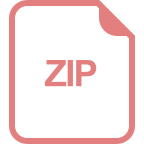
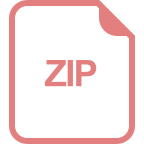













