根据c语言知识,完成下列完整代码:KMP 算法是在已知模式串的next函数值的基础上执行的,那么,如何求得模式串的 next函数值呢?
时间: 2024-11-04 08:11:31 浏览: 25
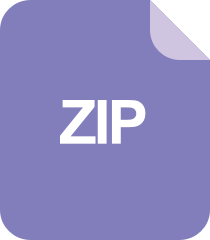
kmp算法-基于C语言实现的kmp模式匹配算法.zip
KMP算法,全称为Knuth-Morris-Pratt算法,用于在一个文本字符串中查找指定的模式串。next函数值的计算主要是为了处理前缀函数,以便于避免无效匹配。下面是一个简单的C语言版本的KMP算法,包括计算next函数的过程:
```c
#include <stdio.h>
#include <string.h>
// 计算next数组
void computeNext(char* pattern, int* next) {
int len = strlen(pattern);
next[0] = -1; // 初始化首个元素为-1表示没有前缀
int j = -1; // 上一个匹配的位置
for (int i = 1; i < len; ++i) {
while (j != -1 && pattern[i] != pattern[j + 1]) {
j = next[j]; // 如果当前字符与前缀不符,回溯到下一个可能的起点
}
if (pattern[i] == pattern[j + 1]) {
j++; // 同样的字符,继续向前推进
}
next[i] = j; // 记录当前位置的前缀长度
}
}
// KMP搜索主函数
int kmpSearch(char* text, char* pattern) {
int textLen = strlen(text), patternLen = strlen(pattern);
int* next = (int*)malloc(sizeof(int) * patternLen); // 存储next函数值
computeNext(pattern, next);
int matchIndex = 0;
for (int i = 0; i < textLen; ++i) {
if (text[i] == pattern[matchIndex]) {
matchIndex++;
if (matchIndex == patternLen) {
printf("Pattern found at index %d\n", i - patternLen + 1);
matchIndex = next[matchIndex - 1];
}
} else if (matchIndex > 0) {
matchIndex = next[matchIndex - 1];
}
}
free(next); // 释放内存
return matchIndex == 0; // 返回是否找到模式串
}
int main() {
char text[] = "ababcababa";
char pattern[] = "ababa";
if (!kmpSearch(text, pattern)) {
printf("Pattern not found.\n");
}
return 0;
}
```
在这个例子中,`computeNext`函数负责计算模式串`pattern`的next函数值,`kmpSearch`函数则是实际的搜索过程,它利用next数组在`text`中查找`pattern`。
阅读全文
相关推荐
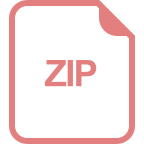
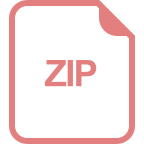

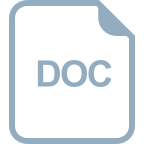
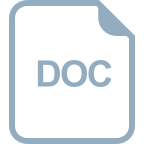
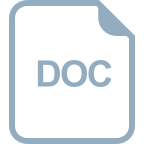
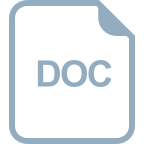
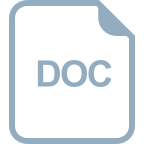
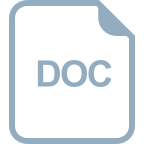








