如果目标是一个矩形,找到这个矩形的任意一点都算成功,怎么处理
时间: 2024-04-14 20:30:20 浏览: 14
如果目标是一个矩形,而不是一个具体的点,那么可以进行以下修改来处理:
1. 将 `goal_node` 更改为一个矩形的信息,例如左上角和右下角的坐标。
2. 在 A* 算法的终止条件中,将目标节点的位置是否在当前节点所代表的矩形范围内作为判断条件之一。这样,只要最终节点在目标矩形范围内,就算成功找到路径。
以下是修改后的代码示例:
```python
import heapq
class Node:
def __init__(self, position, parent=None):
self.position = position
self.parent = parent
self.g = 0 # Cost from start node to current node
self.h = 0 # Heuristic cost from current node to goal node
self.f = 0 # Total cost
def __lt__(self, other):
return self.f < other.f
def astar(grid, start, goal_rect):
open_list = []
closed_list = set()
start_node = Node(start)
goal_node = Node(None)
goal_node.position = get_goal_position(grid, goal_rect)
heapq.heappush(open_list, start_node)
while open_list:
current_node = heapq.heappop(open_list)
closed_list.add(current_node.position)
if is_position_in_rect(current_node.position, goal_rect):
path = []
while current_node.parent:
path.append(current_node.position)
current_node = current_node.parent
path.append(start_node.position)
return path[::-1]
neighbors = get_neighbors(grid, current_node)
for neighbor in neighbors:
if neighbor.position in closed_list:
continue
cost = current_node.g + 1
if neighbor not in open_list or cost < neighbor.g:
neighbor.g = cost
neighbor.h = heuristic(neighbor, goal_node)
neighbor.f = neighbor.g + neighbor.h
neighbor.parent = current_node
if neighbor not in open_list:
heapq.heappush(open_list, neighbor)
return None
def get_neighbors(grid, node):
neighbors = []
directions = [(0, 1), (0, -1), (1, 0), (-1, 0)] # Up, down, left, right
for dir in directions:
new_position = (node.position[0] + dir[0], node.position[1] + dir[1])
if is_valid_position(grid, new_position):
neighbors.append(Node(new_position, node))
return neighbors
def is_valid_position(grid, position):
rows = len(grid)
cols = len(grid[0])
return 0 <= position[0] < rows and 0 <= position[1] < cols and grid[position[0]][position[1]] == 0
def heuristic(node, goal_node):
return abs(node.position[0] - goal_node.position[0]) + abs(node.position[1] - goal_node.position[1])
def get_goal_position(grid, goal_rect):
for row in range(len(grid)):
for col in range(len(grid[0])):
if is_position_in_rect((row, col), goal_rect):
return (row, col)
def is_position_in_rect(position, rect):
rect_x1, rect_y1, rect_x2, rect_y2 = rect
x, y = position
return rect_x1 <= x <= rect_x2 and rect_y1 <= y <= rect_y2
# 测试示例
grid = [
[0, 0, 0, 0, 0],
[0, 1, 0, 1, 0],
[0, 0, 0, 1, 0],
[0, 1, 0, 0, 0],
[0, 0, 0, 0, 0]
]
start = (0, 0)
goal_rect = (1, 1, 3, 3) # 矩形的左上角和右下角坐标
path = astar(grid, start, goal_rect)
if path:
print("Path found:")
for position in path:
print(position)
else:
print("No path found.")
```
在这个修改后的代码中,`goal_rect` 表示目标矩形的左上角和右下角坐标。`get_goal_position` 函数用于找到目标矩形内的任意一点作为目标节点。然后,`is_position_in_rect` 函数用于判断一个节点是否在目标矩形内。
这样,当最终节点在目标矩形内时,就算成功找到路径。你可以根据需要对代码进行进一步的修改和扩展。
相关推荐
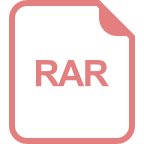
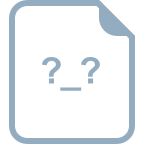
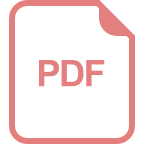














