请用java编写:计算两个同为三阶矩阵的相加、相减、相乘的结果(矩阵中的数随机产生)。
时间: 2024-03-17 18:47:39 浏览: 56
以下是Java代码:
```java
import java.util.Random;
public class Matrix {
private int[][] matrix;
private int row;
private int col;
public Matrix(int row, int col) {
this.row = row;
this.col = col;
matrix = new int[row][col];
Random rand = new Random();
for(int i = 0; i < row; i++) {
for(int j = 0; j < col; j++) {
matrix[i][j] = rand.nextInt(10); // 产生0~9之间的随机数
}
}
}
public void print() {
for(int i = 0; i < row; i++) {
for(int j = 0; j < col; j++) {
System.out.print(matrix[i][j] + " ");
}
System.out.println();
}
}
public Matrix add(Matrix other) {
if(this.row != other.row || this.col != other.col) {
System.out.println("两个矩阵的行列数不相等,不能相加");
return null;
}
Matrix result = new Matrix(row, col);
for(int i = 0; i < row; i++) {
for(int j = 0; j < col; j++) {
result.matrix[i][j] = this.matrix[i][j] + other.matrix[i][j];
}
}
return result;
}
public Matrix subtract(Matrix other) {
if(this.row != other.row || this.col != other.col) {
System.out.println("两个矩阵的行列数不相等,不能相减");
return null;
}
Matrix result = new Matrix(row, col);
for(int i = 0; i < row; i++) {
for(int j = 0; j < col; j++) {
result.matrix[i][j] = this.matrix[i][j] - other.matrix[i][j];
}
}
return result;
}
public Matrix multiply(Matrix other) {
if(this.col != other.row) {
System.out.println("第一个矩阵的列数不等于第二个矩阵的行数,不能相乘");
return null;
}
Matrix result = new Matrix(this.row, other.col);
for(int i = 0; i < this.row; i++) {
for(int j = 0; j < other.col; j++) {
for(int k = 0; k < this.col; k++) {
result.matrix[i][j] += this.matrix[i][k] * other.matrix[k][j];
}
}
}
return result;
}
public static void main(String[] args) {
Matrix a = new Matrix(3, 3);
System.out.println("矩阵a:");
a.print();
Matrix b = new Matrix(3, 3);
System.out.println("矩阵b:");
b.print();
Matrix c = a.add(b);
System.out.println("矩阵a+b:");
c.print();
Matrix d = a.subtract(b);
System.out.println("矩阵a-b:");
d.print();
Matrix e = a.multiply(b);
System.out.println("矩阵a*b:");
e.print();
}
}
```
与前面的代码相比,这段代码没有对矩阵的行和列数进行硬编码,而是在 main 方法中创建了两个 3x3 的矩阵 a 和 b,然后计算了它们的和、差、积,并打印出来。
阅读全文
相关推荐


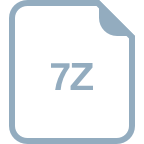
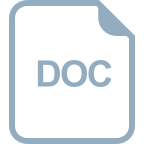
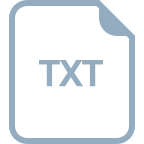
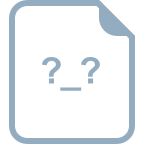
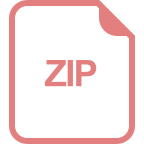
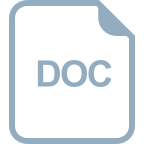





