构建一个包含残差结构的VGG网络,使用pytorch实现
时间: 2023-03-02 07:04:57 浏览: 78
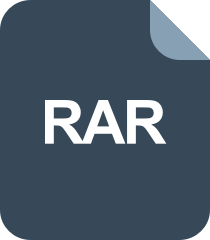
pytorch实现VGG网络
下面是在PyTorch中实现带有残差结构的VGG网络的代码示例:
```
import torch
import torch.nn as nn
import torch.nn.functional as F
class ResidualVGG(nn.Module):
def __init__(self, in_channels, out_channels, stride=1):
super(ResidualVGG, self).__init__()
self.conv1 = nn.Conv2d(in_channels, out_channels, 3, stride=stride, padding=1)
self.bn1 = nn.BatchNorm2d(out_channels)
self.conv2 = nn.Conv2d(out_channels, out_channels, 3, stride=1, padding=1)
self.bn2 = nn.BatchNorm2d(out_channels)
self.stride = stride
def forward(self, x):
residual = x
out = F.relu(self.bn1(self.conv1(x)))
out = self.bn2(self.conv2(out))
if self.stride != 1 or x.shape[1] != out.shape[1]:
residual = F.avg_pool2d(x, 2, stride=2)
out += residual
out = F.relu(out)
return out
class VGGNet(nn.Module):
def __init__(self, num_classes=1000):
super(VGGNet, self).__init__()
self.conv1 = nn.Conv2d(3, 64, 3, stride=1, padding=1)
self.bn1 = nn.BatchNorm2d(64)
self.layer1 = self._make_layer(64, 128, 2)
self.layer2 = self._make_layer(128, 256, 2)
self.layer3 = self._make_layer(256, 512, 2)
self.layer4 = self._make_layer(512, 512, 2)
self.fc = nn.Linear(512, num_classes)
def _make_layer(self, in_channels, out_channels, num_blocks, stride=1):
layers = []
for i in range(num_blocks):
if i == 0:
layers.append(ResidualVGG(in_channels, out_channels, stride))
else:
layers.append(ResidualVGG(out_channels, out_channels, stride=1))
return nn.Sequential(*layers)
def forward(self, x):
out = F.relu(self.bn1(self.conv1(x)))
out = self.layer1(out)
out = self.layer2(out)
out = self.layer3(out)
out = self.layer4(out)
out = F.avg_pool2d(out, 4)
out = out.view(out.size
阅读全文
相关推荐



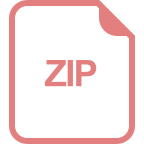
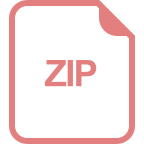



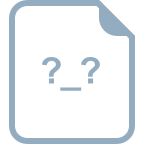
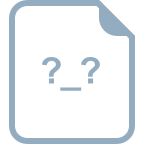
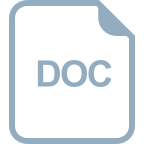
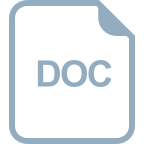
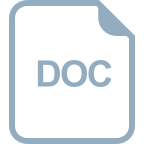


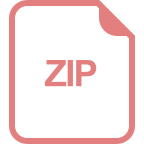