用python写一个打地鼠的小游戏
时间: 2024-12-08 18:16:21 浏览: 13
在Python中编写一个简单的打地鼠小游戏可以使用pygame库,这是一个流行的用于制作2D游戏的框架。下面是一个基础的示例,展示如何创建基本的游戏环境和玩家击打动作:
```python
import pygame
import random
# 初始化pygame
pygame.init()
# 设置窗口大小
screen_width = 800
screen_height = 600
screen = pygame.display.set_mode((screen_width, screen_height))
pygame.display.set_caption("简单打地鼠游戏")
# 地鼠位置
mole_positions = [(random.randint(50, 750), random.randint(50, 550)) for _ in range(3)]
# 游戏循环
done = False
clock = pygame.time.Clock()
while not done:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT or (event.type == pygame.KEYDOWN and event.key == pygame.K_ESCAPE):
done = True
# 渲染背景
screen.fill((255, 255, 255))
# 移动鼠标
mouse_pos = pygame.mouse.get_pos()
# 检查玩家是否点击了地鼠
for i, mole in enumerate(mole_positions):
pygame.draw.circle(screen, (255, 0, 0), mole, 20) # 红色地鼠圆圈
if pygame.Rect(mouse_pos, (20, 20)).colliderect(pygame.Rect(*mole, 40)): # 检测碰撞
print(f"击中第{i+1}只地鼠!")
mole_positions.pop(i)
# 更新屏幕
pygame.display.flip()
# 控制帧率
clock.tick(60)
# 关闭窗口
pygame.quit()
```
这个例子只是一个非常简化的版本,实际的游戏可能会有更复杂的逻辑,如计分系统、隐藏的地鼠以及游戏结束条件等。你可以根据需要对其进行扩展。
阅读全文
相关推荐
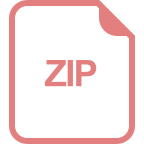
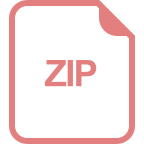
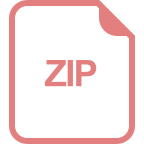






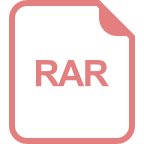







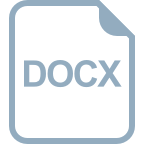