python中for each p in
时间: 2024-04-24 09:25:37 浏览: 60
Python中的for循环语法是使用关键字`for`,后面接一个迭代变量,再加上关键字`in`和一个可迭代对象。如果你想使用类似"for each p in"的语法,可以这样写:
```python
for p in iterable:
# 在这里执行循环体的代码
```
其中,`iterable`是一个可迭代的对象,例如列表、元组、集合或者字符串。每次循环时,迭代变量`p`会依次取到可迭代对象中的元素,并执行循环体中的代码。你可以根据具体需求来替换`iterable`和循环体的代码。
相关问题
Input: CPoints, i.e., the candidate points of the QTS Output: Ppoints, i.e., the period points used to split the QTS. 1. G ¼ getGraph(CPoints); // turns CPoints into graph G 2. COM ¼ convert(G); // concert G into communities COM 3. for each community in COM, denoted as COMi do 4. for each community in COM other than COMi, denoted as COMj do 5. tentatively move COMi into COMj 6. if the modularity of COM is not improved then 7. revoke the above move 8. end if 9. end for 10. end for 11. if some communities were successively moved then 12. go to line 3 to start other round of movement 13. end if 14. F ¼ getFeature(COM); // compute the features of each community 15. C’ ¼ k-means(COM,F); // cluster COM using k-means based on F 16. t ¼ getThreshold(C’); // compute the threshold t based on C’. 17. for each element of F, denoted as Fi do 18. if Fi < t then 19. remove COMi from COM, where Fi is derived from COMi 20. end if 21. end for 22. AMC ¼ getAMC(COM); // compute the AMC of each community 23. AMCmax ¼ getMAX(AMC); // get the maximum AMC 24. PPoints ¼ vertexes in the community corresponding to AMCmax;转换为python代码
以下是该算法的 Python 实现:
```python
from networkx.algorithms.community import greedy_modularity_communities
from sklearn.cluster import KMeans
def getGraph(CPoints):
# 将 CPoints 转换为 networkx 的图形式
pass
def convert(G):
# 将图 G 划分为社区
return list(greedy_modularity_communities(G))
def getFeature(COM):
# 计算每个社区的特征值
pass
def getThreshold(C):
# 根据聚类结果计算阈值
pass
def getAMC(COM):
# 计算每个社区的 AMC
pass
def getMAX(AMC):
# 找到 AMC 的最大值
pass
def splitQTS(CPoints):
G = getGraph(CPoints)
COM = convert(G)
while True:
flag = False
for i, COMi in enumerate(COM):
for j, COMj in enumerate(COM):
if i != j:
COM_new = COM.copy()
COM_new[j].update(COMi)
COM_new[i] = set()
modularity_new = modularity(G, COM_new)
if modularity_new > modularity(G, COM):
COM = COM_new
flag = True
break
if flag:
break
if not flag:
break
F = getFeature(COM)
C = KMeans(n_clusters=3).fit(COM, F).labels_
t = getThreshold(C)
for i, Fi in enumerate(F):
if Fi < t:
COM[i] = set()
AMC = getAMC(COM)
AMCmax = getMAX(AMC)
PPoints = [v for v in COM[AMC.index(AMCmax)]]
return PPoints
```
其中,`modularity` 是计算模块度的函数,需要根据具体情况实现。其他函数的实现方式也需要根据具体的要求来完成。
14. F ¼ getFeature(COM); // compute the features of each community 15. C’ ¼ k-means(COM,F); // cluster COM using k-means based on F 16. t ¼ getThreshold(C’); // compute the threshold t based on C’. 17. for each element of F, denoted as Fi do 18. if Fi < t then 19. remove COMi from COM, where Fi is derived from COMi 20. end if 21. end for 22. AMC ¼ getAMC(COM); // compute the AMC of each community 23. AMCmax ¼ getMAX(AMC); // get the maximum AMC 24. PPoints ¼ vertexes in the community corresponding to AMCmax;翻译成python代码
这段伪代码的主要功能是对社区进行聚类,并计算每个社区的特征和 AMC,然后根据 AMC 的最大值来选择一个社区。下面是一个示例 Python 代码来实现这个伪代码:
```python
from sklearn.cluster import KMeans
def getFeature(COM):
# 计算每个社区的特征
F = []
# ...
return F
def getThreshold(C):
# 根据聚类结果计算阈值
t = 0
# ...
return t
def removeCommunity(COM, F, t):
# 根据阈值移除一些社区
for i in range(len(F)):
if F[i] < t:
del COM[i]
del F[i]
def getAMC(COM):
# 计算每个社区的 AMC
AMC = []
# ...
return AMC
def getMax(AMC):
# 获取最大 AMC
return max(AMC)
def main(COM):
# compute the features of each community
F = getFeature(COM)
# cluster COM using k-means based on F
kmeans = KMeans(n_clusters=4).fit(F)
C_ = kmeans.labels_
# compute the threshold t based on C’
t = getThreshold(C_)
# remove some communities based on threshold t
removeCommunity(COM, F, t)
# compute the AMC of each community
AMC = getAMC(COM)
# get the maximum AMC
AMCmax = getMax(AMC)
# get the vertexes in the community corresponding to AMCmax
PPoints = []
for i in range(len(AMC)):
if AMC[i] == AMCmax:
PPoints.append(COM[i])
return PPoints
```
需要注意的是,这只是一种示例代码,实际应用中需要根据具体情况进行修改和优化。
阅读全文
相关推荐
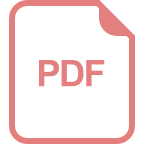
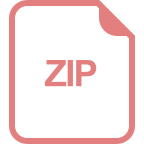
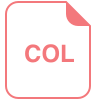
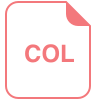
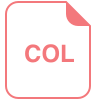
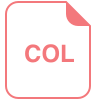
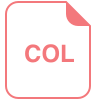
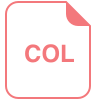








