已知带头节点的循环链表L中至少有两个节点,每个结点的两个域为data和next,其中data的类型为整形,设计一个算法判断该链表中的每个结点值是否小于它后面两个结点值之和。如果满足,返回true,否则返回false。用C语言编写
时间: 2024-09-29 10:05:10 浏览: 29
为了判断一个带头节点的循环链表中每个节点的数据是否小于其后两个节点的数据之和,我们可以遍历整个链表,并维护当前节点、下一个节点以及再下一个节点的信息。以下是使用C语言的一个基本思路:
```c
#include <stdbool.h>
// 定义链表节点结构
typedef struct ListNode {
int data;
struct ListNode* next;
} ListNode;
bool isLessThanSum(ListNode* head) {
if (head == NULL || head->next == NULL || head->next->next == NULL) {
// 如果链表少于3个节点,则无法判断条件,直接返回false
return false;
}
ListNode *current = head;
bool foundCycle = false; // 标记是否找到环
// 使用快慢指针寻找循环点
ListNode *slow = current;
ListNode *fast = current->next->next;
while (fast != slow && fast != NULL && fast->next != NULL) {
slow = slow->next;
fast = fast->next->next;
foundCycle = true;
}
// 如果找到环,将快指针移到头节点,继续遍历
if (foundCycle) {
fast = head;
}
// 遍历链表,检查条件
while (current != fast) {
if (current->data >= current->next->data + current->next->next->data) {
// 如果当前节点值大于等于后两个节点值之和,返回false
return false;
}
current = current->next;
if (current == fast) { // 如果到达环内节点,退出循环
break;
}
}
// 检查环内的节点也满足条件
if (current->data < current->next->data + current->next->next->data) {
return false;
}
// 所有节点都满足条件,返回true
return true;
}
```
这个算法首先检查链表长度,若长度不足3则无法判断。然后使用快慢指针找出循环点。接着从循环开始处遍历链表,检查每个节点的条件。如果找到任何一个节点不满足条件,就返回false;遍历完整个链表(包括环内的节点)都没发现问题,则返回true。
阅读全文
相关推荐
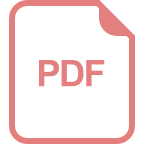
















