opengl绘制文字
时间: 2023-07-31 07:05:10 浏览: 315
在OpenGL中绘制文字可以使用以下两种方法:
1. 使用纹理贴图绘制文字:将每个字符渲染到一个独立的纹理上,然后在OpenGL中使用纹理贴图的方式绘制文字。这种方法的优点是可以实现高质量的字体渲染效果,而且可以对每个字符进行单独的变换和动画效果。缺点是需要加载大量的纹理数据,对内存和显存的要求比较高。
2. 使用位图字体绘制文字:将所有的字符渲染到一个位图上,然后在OpenGL中使用纹理贴图的方式绘制文字。这种方法的优点是比较简单,只需要加载一个纹理,就可以实现绘制所有的字符。缺点是字体质量比较低,而且不能对单个字符进行变换和动画效果。
其中第二种方法比较简单,下面是示例代码:
1. 加载位图字体纹理
```c++
GLuint fontTexture;
glGenTextures(1, &fontTexture);
glBindTexture(GL_TEXTURE_2D, fontTexture);
// 加载位图字体数据
unsigned char *bitmapData = loadBitmapData("font.bmp");
glTexImage2D(GL_TEXTURE_2D, 0, GL_RGBA, bitmapWidth, bitmapHeight, 0, GL_RGBA, GL_UNSIGNED_BYTE, bitmapData);
// 设置纹理参数
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_S, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_WRAP_T, GL_CLAMP_TO_EDGE);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MIN_FILTER, GL_LINEAR);
glTexParameteri(GL_TEXTURE_2D, GL_TEXTURE_MAG_FILTER, GL_LINEAR);
```
2. 绘制文字
```c++
void drawText(const char *text, float x, float y, float size)
{
glEnable(GL_TEXTURE_2D);
glBindTexture(GL_TEXTURE_2D, fontTexture);
glMatrixMode(GL_PROJECTION);
glPushMatrix();
glLoadIdentity();
glOrtho(0, width, 0, height, -1, 1);
glMatrixMode(GL_MODELVIEW);
glPushMatrix();
glLoadIdentity();
glTranslatef(x, y, 0);
glScalef(size, size, 1);
const int stride = 32;
const int numChars = 96;
for (const char *p = text; *p; p++) {
int c = *p - 32;
float tx = (c % stride) / (float)stride;
float ty = (c / stride) / (float)stride;
glBegin(GL_QUADS);
glTexCoord2f(tx, ty + 1.0f/stride); glVertex2f(0, 0);
glTexCoord2f(tx + 1.0f/stride, ty + 1.0f/stride); glVertex2f(1, 0);
glTexCoord2f(tx + 1.0f/stride, ty); glVertex2f(1, 1);
glTexCoord2f(tx, ty); glVertex2f(0, 1);
glEnd();
glTranslatef(0.6f, 0, 0);
}
glPopMatrix();
glMatrixMode(GL_PROJECTION);
glPopMatrix();
glMatrixMode(GL_MODELVIEW);
}
```
以上代码中,`loadBitmapData()`函数用于加载位图字体数据,`width`和`height`表示屏幕的宽度和高度,`drawText()`函数用于绘制文字。在`drawText()`函数中,首先启用纹理贴图并绑定位图字体纹理,然后设置投影矩阵和模型视图矩阵,根据字符的ASCII码计算对应的纹理坐标,使用`glBegin()`和`glEnd()`函数绘制一个矩形,最后向右平移一定距离,绘制下一个字符。
阅读全文
相关推荐
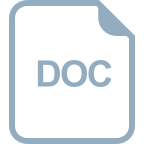
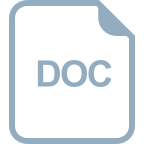
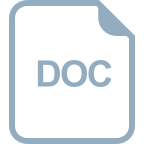

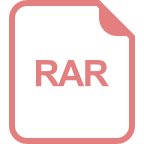
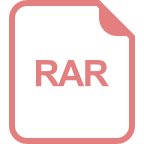
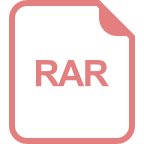
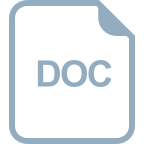
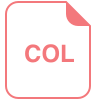





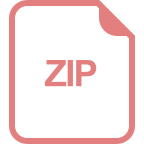
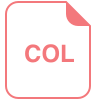
