计算链表长度,c语言,包含链表的建立以及main函数
时间: 2024-11-13 15:12:46 浏览: 8
在C语言中,计算链表长度通常涉及创建链表节点、链接节点、初始化链表以及遍历链表来获取长度。下面是一个简单的例子,包括链表的创建、插入节点、查找长度并在`main`函数中实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
struct Node {
int data;
struct Node* next;
};
// 创建新节点
struct Node* createNode(int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = NULL;
}
return newNode;
}
// 在链表尾部添加节点
void append(struct Node** head, int value) {
if (*head == NULL) {
*head = createNode(value);
} else {
struct Node* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = createNode(value);
}
}
// 计算并返回链表长度
int getLength(struct Node* head) {
int length = 0;
struct Node* temp = head;
while (temp != NULL) {
length++;
temp = temp->next;
}
return length;
}
// 主函数
int main() {
// 初始化链表为空
struct Node* head = NULL;
// 插入示例数据(这里仅作演示,可根据需要修改)
append(&head, 1);
append(&head, 2);
append(&head, 3);
// 计算链表长度
int listLength = getLength(head);
printf("链表长度: %d\n", listLength);
// 清理内存(仅作为示例,实际应用中根据需求处理)
struct Node* temp;
while (head != NULL) {
temp = head;
head = head->next;
free(temp);
}
return 0;
}
```
在这个例子中,我们首先定义了链表节点的结构,然后创建了用于创建新节点、在链表尾部添加节点和计算链表长度的函数。在`main`函数中,我们初始化了一个空链表,并插入了一些元素,然后通过`getLength`函数获取链表长度并打印出来。
阅读全文
相关推荐
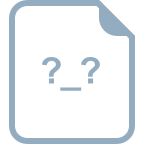
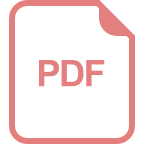
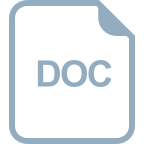



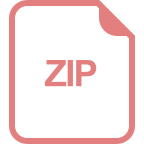
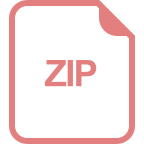
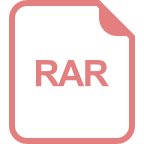
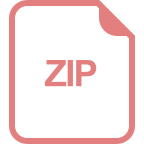
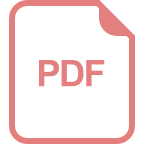
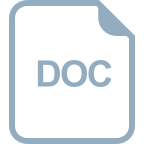






