使用文件操作函数,编程实现cp命令的功能。(提示:open read write close)
时间: 2024-10-25 10:08:13 浏览: 14
在编程中,实现类似于 `cp` 命令的功能通常涉及以下几个步骤,使用 Python 的 `os` 和 `shutil` 模块为例:
1. **打开源文件**:
使用 `os.open()` 或者 `shutil.copyfileobj()` 函数打开源文件,如果需要读取文件内容,则以只读模式 (`os.O_RDONLY`) 打开。
```python
import os
src_path = 'source.txt'
src_file = os.open(src_path, os.O_RDONLY)
```
2. **创建目标文件**:
如果目标文件不存在,使用 `os.mkfifo()` 或 `open()` 创建一个新的文件,如果是复制整个目录,则可以使用 `shutil.copytree()`。
```python
dst_path = 'destination.txt'
if not os.path.exists(dst_path):
if os.path.isdir(src_path): # 如果是目录
shutil.copytree(src_path, dst_path)
else: # 如果是文件
dst_file = open(dst_path, 'w')
```
3. **读取并写入文件内容**:
对于文件,可以使用 `os.read()` 逐块读取源文件的内容,并写入到目标文件。
```python
buf_size = 4096
data = b''
while True:
data = os.read(src_file, buf_size)
if not data:
break
dst_file.write(data)
dst_file.flush()
```
4. **关闭文件**:
最后,记得关闭打开的所有文件。
```python
os.close(src_file)
if isinstance(dst_file, int): # 如果是文件描述符
os.close(dst_file)
else:
dst_file.close()
```
阅读全文
相关推荐
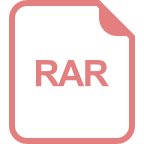
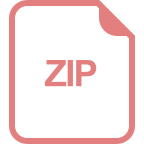
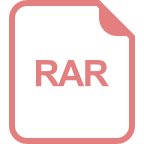















