verilog fifo代码
时间: 2023-07-29 20:08:39 浏览: 87
Sure! Here's an example of a Verilog code for a simple FIFO (First-In-First-Out) implementation:
```verilog
module fifo(
input wire clk, // Clock signal
input wire rst, // Reset signal
input wire wr_en, // Write enable signal
input wire rd_en, // Read enable signal
input wire [7:0] data_in, // Input data
output wire [7:0] data_out // Output data
);
parameter DEPTH = 16; // Depth of the FIFO
reg [7:0] mem [DEPTH-1:0]; // Memory array for storing data
reg [3:0] wr_ptr; // Write pointer
reg [3:0] rd_ptr; // Read pointer
reg [3:0] count; // Number of elements in the FIFO
always @(posedge clk or posedge rst) begin
if (rst) begin
wr_ptr <= 0;
rd_ptr <= 0;
count <= 0;
end else begin
if (wr_en && count < DEPTH) begin
mem[wr_ptr] <= data_in;
wr_ptr <= wr_ptr + 1;
count <= count + 1;
end
if (rd_en && count > 0) begin
data_out <= mem[rd_ptr];
rd_ptr <= rd_ptr + 1;
count <= count - 1;
end
end
end
endmodule
```
In this code, the FIFO module has clock (`clk`), reset (`rst`), write enable (`wr_en`), read enable (`rd_en`), input data (`data_in`), and output data (`data_out`) signals. It uses a parameter `DEPTH` to specify the depth of the FIFO, which is set to 16 in this example.
The FIFO is implemented using a memory array `mem` to store the data, along with write and read pointers `wr_ptr` and `rd_ptr`, and a count of elements `count` in the FIFO.
The `always @(posedge clk or posedge rst)` block is used to synchronize the operations with the clock signal. On a positive clock edge or reset, the write pointer, read pointer, and count are reset.
During normal operation, when the write enable signal is active (`wr_en` is high) and there is space available in the FIFO (`count < DEPTH`), data is written into the memory array at the current write pointer location. The write pointer and count are incremented accordingly.
Similarly, when the read enable signal is active (`rd_en` is high) and there are elements in the FIFO (`count > 0`), data is read from the memory array at the current read pointer location. The read pointer and count are updated accordingly.
Note that this is a simple example of a Verilog code for a FIFO and can be further enhanced or modified based on specific requirements.
阅读全文
相关推荐



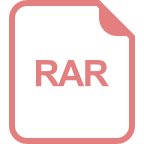
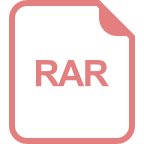
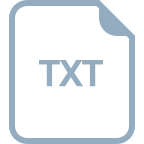
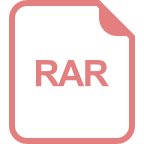
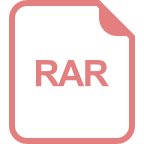
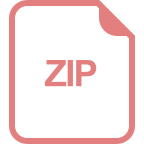
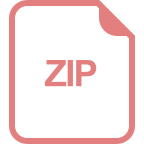
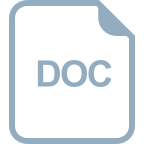
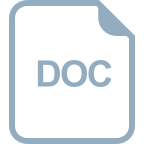
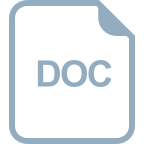
