如何在C++中实现圆柱体积计算?
时间: 2024-10-20 13:14:11 浏览: 12
在C++中实现圆柱体积计算,你可以参考以下代码:
```cpp
#include <iostream>
#include <cmath> // 引入数学库以使用PI和pow函数
const double PI = 3.14159; // 定义常量PI
double calculate_cylinder_volume(double radius, double height) {
return PI * std::pow(radius, 2) * height; // 使用 pow 函数计算半径的平方
}
int main() {
double r, h;
std::cout << "请输入圆柱体的半径:";
std::cin >> r;
std::cout << "请输入圆柱体的高度:";
std::cin >> h;
double volume = calculate_cylinder_volume(r, h);
std::cout << "圆柱体的体积为:" << volume << "\n"; // 输出体积
return 0;
}
```
这里我们使用了 `<cmath>` 库的 `std::pow` 函数来计算半径的平方,其余部分与之前C语言版本的代码相似。用户通过输入半径和高度,然后调用 `calculate_cylinder_volume` 函数来获取体积。
相关问题
C++实现计算圆柱体积
在C++中,计算圆柱体体积的公式是π * 半径^2 * 高度。下面是一个简单的函数示例:
```cpp
#include <iostream>
#include <cmath> // 引入math库,用于获取π的值
// 定义计算圆柱体积的函数
double calculate_cylinder_volume(double radius, double height) {
const double pi = 3.14159; // 使用近似值表示π
return pi * pow(radius, 2) * height;
}
int main() {
double radius; // 圆柱半径
double height; // 圆柱高度
std::cout << "请输入圆柱的半径: ";
std::cin >> radius;
std::cout << "请输入圆柱的高度: ";
std::cin >> height;
double volume = calculate_cylinder_volume(radius, height);
std::cout << "圆柱的体积为: " << volume << " 立方单位" << std::endl;
return 0;
}
```
在这个程序中,用户输入圆柱的半径和高度,然后调用`calculate_cylinder_volume`函数计算并输出体积。注意,这里假设输入的是双精度浮点数,并使用了`pow`函数来进行平方运算。
c++中定义一个圆柱类,计算圆柱的面积和体积,要求用友元函数来求圆柱的面积和体积?
下面是一个简单的圆柱类的定义,其中使用了友元函数来计算圆柱的面积和体积:
```c++
#include <iostream>
using namespace std;
class Cylinder {
private:
float radius;
float height;
public:
Cylinder(float r, float h) : radius(r), height(h) {}
friend float area(Cylinder c);
friend float volume(Cylinder c);
};
float area(Cylinder c) {
return 2 * 3.14 * c.radius * (c.radius + c.height);
}
float volume(Cylinder c) {
return 3.14 * c.radius * c.radius * c.height;
}
int main() {
Cylinder c(3, 5);
cout << "Area: " << area(c) << endl;
cout << "Volume: " << volume(c) << endl;
return 0;
}
```
在上面的代码中,`Cylinder`类有两个私有成员变量`radius`和`height`,并且有一个构造函数来初始化这些变量。`area`和`volume`函数都是友元函数,它们可以访问`Cylinder`类的私有成员变量。在`main`函数中,我们创建一个`Cylinder`对象`c`,并分别调用`area`和`volume`函数来计算圆柱的面积和体积。
阅读全文
相关推荐
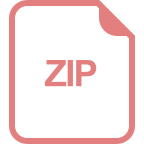
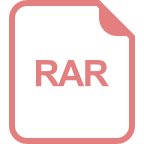
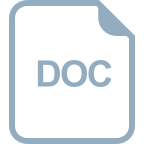
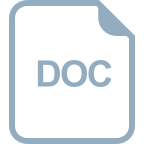


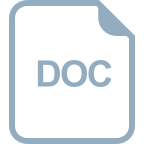
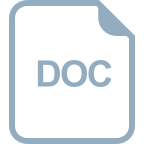






