c++ 求解一元三次方程
时间: 2023-09-04 10:14:17 浏览: 149
要在C++中求解一元三次方程,你可以使用牛顿迭代法或者高斯-赛德尔迭代法等数值方法。以下是一个使用牛顿迭代法求解一元三次方程的示例代码:
```cpp
#include <iostream>
#include <cmath>
double func(double x, double a, double b, double c, double d) {
return a * pow(x, 3) + b * pow(x, 2) + c * x + d;
}
double derivative(double x, double a, double b, double c) {
return 3 * a * pow(x, 2) + 2 * b * x + c;
}
double newtonMethod(double a, double b, double c, double d, double initialGuess, double epsilon, int maxIterations) {
double x = initialGuess;
int iteration = 0;
while (fabs(func(x, a, b, c, d)) > epsilon && iteration < maxIterations) {
x = x - func(x, a, b, c, d) / derivative(x, a, b, c);
iteration++;
}
return x;
}
int main() {
double a, b, c, d;
std::cout << "Enter coefficients a, b, c and d of the cubic equation: ";
std::cin >> a >> b >> c >> d;
double initialGuess;
std::cout << "Enter initial guess for the root: ";
std::cin >> initialGuess;
double epsilon;
std::cout << "Enter epsilon (tolerance): ";
std::cin >> epsilon;
int maxIterations;
std::cout << "Enter maximum number of iterations: ";
std::cin >> maxIterations;
double root = newtonMethod(a, b, c, d, initialGuess, epsilon, maxIterations);
std::cout << "Root: " << root << std::endl;
return 0;
}
```
在此示例中,我们使用牛顿迭代法来寻找方程的根。用户需要输入方程的系数a、b、c、d,以及初始猜测值、容差和最大迭代次数。程序将输出找到的根。
请注意,这只是一个简单的示例,并且不一定适用于所有情况。在实际应用中,您可能需要考虑处理方程无解或多个解的情况,并对算法进行更多的优化和错误处理。
阅读全文
相关推荐
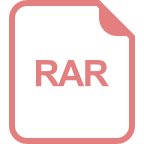
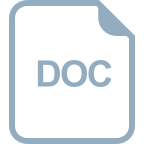







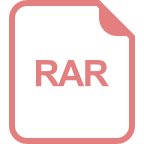
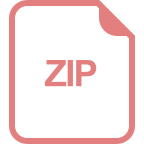
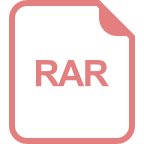



