认真设计一元多项式加法运算的算法
时间: 2023-12-02 15:38:27 浏览: 27
好的,针对一元多项式加法运算,我们可以设计如下算法:
1. 首先将两个多项式按照指数从高到低排列,方便后续的加法运算。
2. 定义一个新的多项式,用于存储相加后的结果。
3. 从两个多项式的最高次项开始,依次将系数相加,并将结果存入新的多项式中。
4. 如果其中一个多项式已经加完了,则将另一个多项式剩余的项直接添加到新的多项式中。
5. 最后得到的新的多项式即为两个多项式相加的结果。
下面是一个 Python 实现的例子:
```python
class Polynomial:
def __init__(self, coeffs):
self.coeffs = coeffs
def __add__(self, other):
result_coeffs = []
i, j = 0, 0
while i < len(self.coeffs) and j < len(other.coeffs):
if self.coeffs[i][0] == other.coeffs[j][0]:
result_coeffs.append((self.coeffs[i][0], self.coeffs[i][1] + other.coeffs[j][1]))
i += 1
j += 1
elif self.coeffs[i][0] > other.coeffs[j][0]:
result_coeffs.append(self.coeffs[i])
i += 1
else:
result_coeffs.append(other.coeffs[j])
j += 1
while i < len(self.coeffs):
result_coeffs.append(self.coeffs[i])
i += 1
while j < len(other.coeffs):
result_coeffs.append(other.coeffs[j])
j += 1
return Polynomial(result_coeffs)
```
其中,`coeffs` 是一个列表,存储了多项式的系数和指数,例如 `[(3, 2), (4, 1), (5, 0)]` 表示 $3x^2 + 4x + 5$。
相关推荐
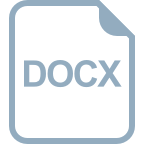
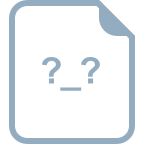














