基于iris数据集,构造二分类决策树模型,尝试使用超参数调优的方式对决策树进行剪枝,并绘制ROC曲线,计算AUC值、预测精度、F1等模型评价指标。要求和第三章构建二分类逻辑回归模型时选择的数据不同。
时间: 2024-11-03 13:21:43 浏览: 57
基于Iris数据集,首先我们需要加载数据并预处理,通常包括特征缩放、编码分类变量(如果需要的话),以及将目标变量分为训练集和测试集。然后,我们可以使用Python的Scikit-learn库来创建一个决策树分类器,比如`DecisionTreeClassifier`。
为了进行超参数调优,我们可以使用GridSearchCV或RandomizedSearchCV,例如:
```python
from sklearn.model_selection import GridSearchCV
from sklearn.tree import DecisionTreeClassifier
# 假设X_train和y_train是我们的训练数据
param_grid = {'max_depth': [3, 5, 7, None],
'min_samples_split': [2, 5, 10],
'min_samples_leaf': [1, 2, 4]}
clf = DecisionTreeClassifier(random_state=42)
grid_search = GridSearchCV(clf, param_grid, cv=5)
grid_search.fit(X_train, y_train)
best_tree = grid_search.best_estimator_
```
接着,我们对训练好的决策树进行剪枝,可以使用`prune()`函数:
```python
from sklearn.tree import prune
pruned_tree = prune(best_tree)
```
评估模型性能时,我们需要先用测试集做预测,然后计算各种指标:
```python
from sklearn.metrics import roc_curve, auc, accuracy_score, f1_score
y_pred_proba = pruned_tree.predict_proba(X_test)[:, 1] # 第一列对应正类概率
fpr, tpr, _ = roc_curve(y_test, y_pred_proba)
roc_auc = auc(fpr, tpr)
accuracy = accuracy_score(y_test, y_pred)
f1 = f1_score(y_test, y_pred, average='weighted') # 可以指定average参数为'micro', 'macro', 'weighted'
plt.figure()
plt.plot(fpr, tpr, label=f'AUC = {roc_auc:.2f}')
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.legend(loc='lower right')
```
这里我们得到了ROC曲线,AUC值、预测精度和F1分数。记得根据实际结果分析模型的表现,看看是否达到了预期的效果,以及是否存在改进的空间。
阅读全文
相关推荐
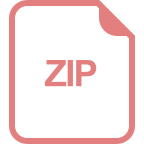
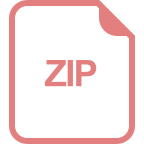
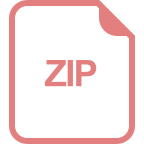
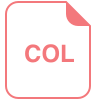
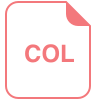
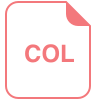
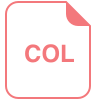
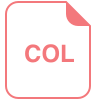
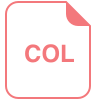
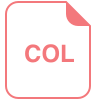
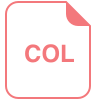
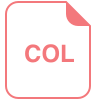
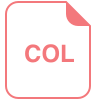
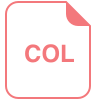
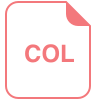
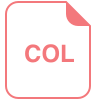
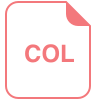
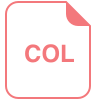