C语言编写一个递归函数,计算二叉树深度。
时间: 2024-11-17 21:29:14 浏览: 33
在C语言中,计算二叉树的深度通常涉及到递归算法。你可以定义一个递归函数`binaryTreeDepth`,其基本思路是从根节点开始,如果当前节点非空,则向左和右子节点递归地求深度,并返回其中的最大值加一。下面是简单的代码示例:
```c
#include <stdio.h>
// 定义二叉树节点结构体
typedef struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
} TreeNode;
// 计算二叉树深度的递归函数
int binaryTreeDepth(TreeNode* root) {
// 如果树为空,深度为0
if (root == NULL) {
return 0;
}
// 否则,左右子树深度加上1即为当前树的深度
int left_depth = binaryTreeDepth(root->left);
int right_depth = binaryTreeDepth(root->right);
// 返回较大的那个子树深度作为当前节点的深度
return (left_depth > right_depth ? left_depth : right_depth) + 1;
}
int main() {
// 创建一个示例二叉树
TreeNode* tree = /* 初始化二叉树... */;
int depth = binaryTreeDepth(tree);
printf("The depth of the binary tree is %d.\n", depth);
return 0;
}
```
在这个例子中,
阅读全文
相关推荐
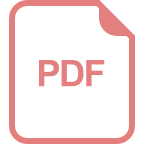
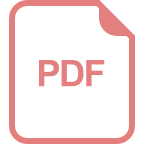
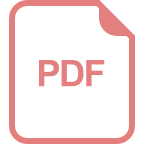

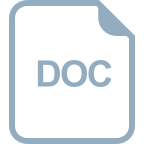
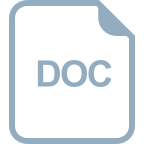












