java编写查找二叉树某个结点的父结点的算法。要求: 1.采用链表存储结构,添加该方法为其成员方法; 2、编写测试程序,创建一棵二叉树,测试查找某个给定结点的父结点。
时间: 2024-12-01 22:29:40 浏览: 10
在Java中,我们可以使用递归的方式来实现二叉树节点查找其父节点的算法。假设我们有一个`BinaryTreeNode`类,它包含`value`(值)、`leftChild`(左孩子)和`rightChild`(右孩子)属性,以及表示父节点的引用`parent`。这里是一个简单的实现:
```java
public class BinaryTreeNode {
int value;
BinaryTreeNode leftChild;
BinaryTreeNode rightChild;
BinaryTreeNode parent; // 父节点
// 添加查找父节点的成员方法
public BinaryTreeNode findParent(int targetValue) {
if (this.value == targetValue) { // 当前节点就是目标节点
return parent; // 返回父节点
} else if (targetValue < this.value && this.leftChild != null) { // 向左子树寻找
return this.leftChild.findParent(targetValue);
} else if (targetValue > this.value && this.rightChild != null) { // 向右子树寻找
return this.rightChild.findParent(targetValue);
}
return null; // 如果没有找到则返回null
}
}
// 测试程序
public class Main {
public static void main(String[] args) {
// 创建一颗二叉树
BinaryTreeNode root = new BinaryTreeNode(5); // 根节点值为5
root.leftChild = new BinaryTreeNode(3); // 左子节点值为3
root.rightChild = new BinaryTreeNode(7); // 右子节点值为7
root.leftChild.parent = root; // 设置根节点为3节点的父节点
root.rightChild.parent = root; // 设置根节点为7节点的父节点
// 查找节点并打印其父节点
BinaryTreeNode nodeToFind = new BinaryTreeNode(3); // 要查找的节点值
BinaryTreeNode parentNode = nodeToFind.findParent(root.value);
System.out.println("节点 " + nodeToFind.value + " 的父节点值为: " + (parentNode != null ? parentNode.value : "null"));
}
}
```
这个例子中,我们首先在主函数中创建了一个二叉树,并设置了每个节点的父节点。然后通过调用`findParent`方法查找指定值的节点的父节点。
阅读全文
相关推荐
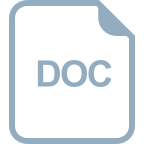
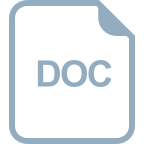
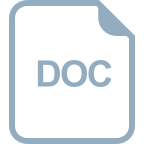












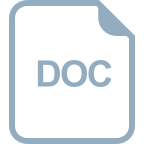
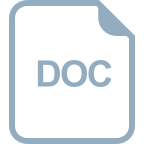
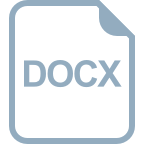