python列表的合并与排序
时间: 2024-01-22 20:02:26 浏览: 68
Python 中的列表可以使用 `+` 运算符进行合并,也可以使用 `extend()` 方法进行合并。例如:
```python
# 合并两个列表
list1 = [1, 2, 3]
list2 = [4, 5, 6]
merged_list = list1 + list2
print(merged_list) # 输出 [1, 2, 3, 4, 5, 6]
# 使用 extend() 方法合并两个列表
list1.extend(list2)
print(list1) # 输出 [1, 2, 3, 4, 5, 6]
```
对于排序,Python 中提供了 `sort()` 方法和 `sorted()` 函数。它们都可以用于对列表进行排序,但是有一些区别:
- `sort()` 方法直接对列表进行排序,不会返回一个新的列表,而是修改原来的列表。
- `sorted()` 函数会返回一个新的排序好的列表,不会修改原来的列表。
例如:
```python
# 使用 sort() 方法对列表进行排序
list1 = [3, 1, 4, 2]
list1.sort()
print(list1) # 输出 [1, 2, 3, 4]
# 使用 sorted() 函数对列表进行排序
list2 = [3, 1, 4, 2]
sorted_list = sorted(list2)
print(sorted_list) # 输出 [1, 2, 3, 4]
print(list2) # 输出 [3, 1, 4, 2]
```
需要注意的是,如果列表中的元素是复杂对象,需要指定排序的 key 函数。例如:
```python
# 对元组列表按照第二个元素排序
list1 = [(1, 3), (2, 1), (3, 4), (4, 2)]
list1.sort(key=lambda x: x[1])
print(list1) # 输出 [(2, 1), (4, 2), (1, 3), (3, 4)]
# 对字典列表按照 age 键排序
list2 = [{'name': 'Alice', 'age': 25}, {'name': 'Bob', 'age': 20}, {'name': 'Charlie', 'age': 30}]
sorted_list = sorted(list2, key=lambda x: x['age'])
print(sorted_list) # 输出 [{'name': 'Bob', 'age': 20}, {'name': 'Alice', 'age': 25}, {'name': 'Charlie', 'age': 30}]
```
阅读全文
相关推荐
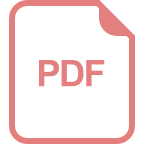

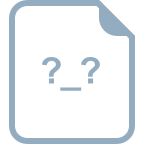
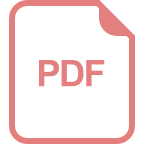
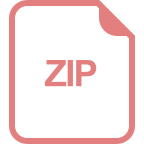
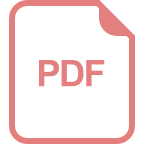
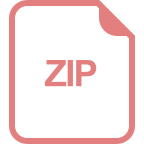
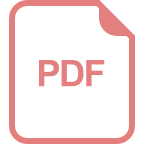
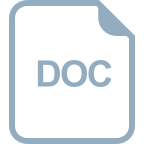





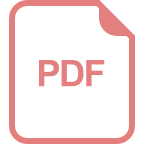