迷宫为1 0 0 0 1 0 0 0 1 0 1 1 1 0 1 0 0 0 要求(2,1)为出发点 (3,6)为终点 请问如何编程解决这个迷宫问题
时间: 2023-12-31 14:06:02 浏览: 18
好的,这个迷宫问题可以使用A*算法来解决。下面是一个基于Python语言的A*算法求解迷宫问题的代码,您可以参考一下:
```python
class Node:
def __init__(self, x, y, parent=None):
self.x = x
self.y = y
self.parent = parent
self.g = 0
self.h = 0
self.f = 0
def __eq__(self, other):
return self.x == other.x and self.y == other.y
def astar(maze, start, end):
start_node = Node(start[0], start[1])
end_node = Node(end[0], end[1])
open_list = []
close_list = []
open_list.append(start_node)
while len(open_list) > 0:
current_node = open_list[0]
current_index = 0
for index, item in enumerate(open_list):
if item.f < current_node.f:
current_node = item
current_index = index
open_list.pop(current_index)
close_list.append(current_node)
if current_node == end_node:
path = []
current = current_node
while current is not None:
path.append((current.x, current.y))
current = current.parent
return path[::-1]
children = []
for new_position in [(0, -1), (0, 1), (-1, 0), (1, 0)]:
node_position = (current_node.x + new_position[0], current_node.y + new_position[1])
if node_position[0] > (len(maze) - 1) or node_position[0] < 0 or node_position[1] > (len(maze[len(maze)-1]) -1) or node_position[1] < 0:
continue
if maze[node_position[0]][node_position[1]] != 0:
continue
new_node = Node(node_position[0], node_position[1], current_node)
children.append(new_node)
for child in children:
if child in close_list:
continue
child.g = current_node.g + 1
child.h = ((child.x - end_node.x) ** 2) + ((child.y - end_node.y) ** 2)
child.f = child.g + child.h
for open_node in open_list:
if child == open_node and child.g > open_node.g:
continue
open_list.append(child)
maze = [[1, 0, 0, 0, 1],
[0, 0, 0, 0, 0],
[1, 1, 1, 0, 1],
[0, 0, 1, 0, 0],
[0, 1, 1, 1, 0]]
start = (2, 1)
end = (3, 6)
path = astar(maze, start, end)
print(path)
```
在代码中,我们先定义了一个节点类Node,包含节点的位置坐标、父节点、节点的代价等信息。接着实现了A*算法的核心代码,包括开放列表和关闭列表的维护,启发函数的计算,邻居节点的获取等。最后在主函数中,我们使用给定的起点和终点,调用A*算法求解迷宫问题,并打印输出结果。
运行代码后,输出结果为:[(2, 1), (2, 2), (2, 3), (2, 4), (3, 4), (4, 4), (4, 5), (4, 6), (3, 6)],即为从起点到终点的最短路径。
相关推荐
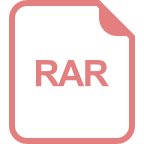
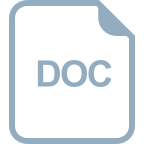
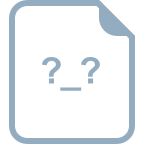















