遗传算法解决多配送中心vrp问题 python代码
时间: 2023-09-14 15:07:47 浏览: 90
以下是使用遗传算法解决多配送中心VRP问题的Python代码示例:
```python
import numpy as np
import random
# 距离矩阵
distance_matrix = np.array([
[0, 3, 1, 5, 8, 6],
[3, 0, 2, 4, 6, 7],
[1, 2, 0, 6, 9, 4],
[5, 4, 6, 0, 3, 1],
[8, 6, 9, 3, 0, 2],
[6, 7, 4, 1, 2, 0]
])
# 需求量
demand = np.array([0, 2, 4, 6, 3, 5])
# 车辆容量限制
capacity = 10
# 遗传算法参数
population_size = 50
elite_size = 10
mutation_rate = 0.01
generations = 100
# 随机生成初始种群
def initial_population(population_size, demand):
population = []
for i in range(population_size):
chromosome = np.random.permutation(len(demand) - 1) + 1
population.append(chromosome)
return population
# 计算染色体的适应度,即路径长度
def fitness(chromosome, distance_matrix, demand, capacity):
path_demand = np.cumsum(demand[chromosome])
path_demand = np.insert(path_demand, 0, 0)
path_demand = np.delete(path_demand, -1)
path_capacity = np.maximum(path_demand, np.roll(path_demand, shift=1))
path_capacity[0] = 0
path_distance = distance_matrix[0, chromosome[0]] + np.sum(distance_matrix[chromosome[:-1], chromosome[1:]]) + distance_matrix[chromosome[-1], 0]
path_penalty = np.sum(np.maximum(path_demand - path_capacity, 0))
if path_penalty > 0 or path_distance > capacity:
return 0
else:
return 1 / path_distance
# 选择精英个体
def select_elite(population, elite_size, distance_matrix, demand, capacity):
fitness_scores = [(fitness(chromosome, distance_matrix, demand, capacity), chromosome) for chromosome in population]
fitness_scores.sort(reverse=True)
elite = []
for i in range(elite_size):
elite.append(fitness_scores[i][1])
return elite
# 交叉操作
def crossover(parent1, parent2):
crossover_point = random.randint(1, len(parent1) - 1)
child1 = np.concatenate((parent1[:crossover_point], [x for x in parent2 if x not in parent1[:crossover_point]]))
child2 = np.concatenate((parent2[:crossover_point], [x for x in parent1 if x not in parent2[:crossover_point]]))
return (child1, child2)
# 变异操作
def mutate(chromosome, mutation_rate):
for i in range(len(chromosome)):
if random.random() < mutation_rate:
j = random.randint(0, len(chromosome) - 1)
chromosome[i], chromosome[j] = chromosome[j], chromosome[i]
return chromosome
# 遗传算法主函数
def genetic_algorithm(distance_matrix, demand, capacity, population_size, elite_size, mutation_rate, generations):
population = initial_population(population_size, demand)
for generation in range(generations):
elite = select_elite(population, elite_size, distance_matrix, demand, capacity)
new_population = elite
while len(new_population) < population_size:
parent1, parent2 = random.sample(elite, 2)
child1, child2 = crossover(parent1, parent2)
child1 = mutate(child1, mutation_rate)
child2 = mutate(child2, mutation_rate)
new_population.append(child1)
new_population.append(child2)
population = new_population
best_chromosome = elite[0]
best_fitness = fitness(best_chromosome, distance_matrix, demand, capacity)
return (best_chromosome, best_fitness)
# 运行遗传算法
best_chromosome, best_fitness = genetic_algorithm(distance_matrix, demand, capacity, population_size, elite_size, mutation_rate, generations)
print('Best Chromosome:', best_chromosome)
print('Best Fitness:', best_fitness)
```
上述代码中的距离矩阵、需求量和车辆容量限制可以根据具体问题进行修改。遗传算法的参数可以根据实际情况进行调整。
相关推荐
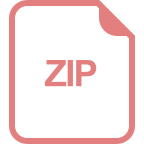
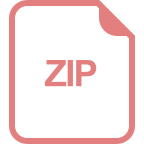














