import matplotlib.pyplot as plt import numpy as np # 构造极坐标数据 r = np.arange(0, 3, 0.002) theta = 2 * np.pi * r # 创建画布和坐标系 fig = plt.figure(figsize=(8, 8)) ax = fig.add_subplot(111, projection='polar') # 绘制螺旋线 ax.plot(theta, r, label='Archimedean spiral') # 设置极坐标刻度 ax.set_rticks([0.5, 1, 1.5, 2, 2.5, 3]) # 计算注释的位置 index = np.argmin((np.pi / 6 - theta) % (0.2*np.pi)) x = theta[index] y = r[index] # 添加文本和注释 ax.annotate('Intersection', xy=(x, y), xytext=(2 * np.pi / 3, 2.5), textcoords='polar', fontsize=12, arrowprops=dict(facecolor='red', arrowstyle='->')) ax.text(2 * np.pi, r[-1], 'End', ha='center', va='center', fontsize=12) # 美化图形 ax.grid(True) line[0].set_color('gray') line[0].set_linestyle('--') ax.spines['polar'].set_visible(False) # 显示图形 plt.show()修改注释的位置使其在相交点上
时间: 2024-01-22 18:02:44 浏览: 141
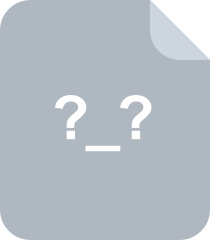
import numpy as np import matplotlib.pyplot as plt import math
要修改注释的位置,只需要将 ax.annotate() 函数中的 xy 参数设为相交点的坐标 (x, y) 即可。同时,还需要适当调整 xytext 参数的值,使得注释框在相交点上方,这样才不会遮挡住曲线。
下面是修改后的代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 构造极坐标数据
r = np.arange(0, 3, 0.002)
theta = 2 * np.pi * r
# 创建画布和坐标系
fig = plt.figure(figsize=(8, 8))
ax = fig.add_subplot(111, projection='polar')
# 绘制螺旋线
ax.plot(theta, r, label='Archimedean spiral')
# 设置极坐标刻度
ax.set_rticks([0.5, 1, 1.5, 2, 2.5, 3])
# 计算注释的位置
index = np.argmin((np.pi / 6 - theta) % (0.2*np.pi))
x = theta[index]
y = r[index]
# 添加文本和注释(修改后)
ax.annotate('Intersection', xy=(x, y), xytext=(x + np.pi / 6, y + 0.5), textcoords='polar', fontsize=12,
arrowprops=dict(facecolor='red', arrowstyle='->'))
# 添加终点文本
ax.text(2 * np.pi, r[-1], 'End', ha='center', va='center', fontsize=12)
# 美化图形
ax.grid(True)
line = ax.get_lines()
line[0].set_color('gray')
line[0].set_linestyle('--')
ax.spines['polar'].set_visible(False)
# 显示图形
plt.show()
```
在上述代码中,我们将 xytext 参数设为相交点坐标 (x + np.pi / 6, y + 0.5),即相交点上方 0.5 的位置。这样调整后,注释框就不会遮挡住曲线,而且也更加清晰易读了。
阅读全文
相关推荐
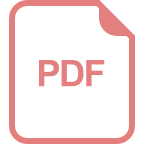











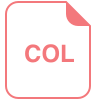
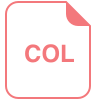
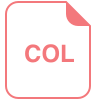
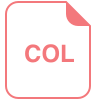
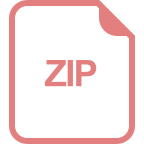