void insertBST(BStree *bst,KeyType K){ BStree s; if(bst==NULL){ s=(BStree)malloc(sizeof(BSTnode)); s->data=K; s->lchild=NULL; s->rchild=NULL; *bst=s; } else if(K<(*bst)->data) insertBST(&((*bst)->lchild),K); else if(K>(*bst)->data) insertBST(&((*bst)->rchild),K); }
时间: 2024-02-19 19:01:44 浏览: 71
这是一个二叉搜索树的插入函数,根据传入的Key值,将其插入到合适的位置上。
函数接收两个参数,第一个是BStree类型的指针,第二个是KeyType类型的值。
首先判断传入的指针是否为空,如果为空,则新建一个节点,并将传入的值赋给该节点,并将该节点赋给传入的指针;如果不为空,则根据传入的值与当前节点的值的大小比较,决定将其插入到该节点的左子树还是右子树中。
该函数采用了递归的方式实现,每次递归时传入的都是当前节点的左/右子树的指针,直到找到一个空指针为止,将新建的节点插入到该位置上。
相关问题
#include<stdio.h> #include<string.h> #include<malloc.h> typedef struct node { int key; struct node *lchild,*rchild; }BSTNode,*BSTree; //二叉排序树插入 void InsertBST(BSTree *bst,int key) { BSTree s; if(*bst==NULL) { s=(BSTree)malloc(sizeof(BSTNode)); s->key=key; s->lchild=NULL; s->rchild=NULL; *bst=s; } } //创建二叉排序树 void CreatBST(BSTree *bst) { int key; *bst=NULL; scanf("%d",&key); while(key!=ENDKEY) { InsertBST(bst,key); scanf("%d",&key); } }填写该代码 使其实现用二叉链表作为存储结构,输入键值序列建立一棵二叉排序树,然后中序遍历这棵二叉树。的功能
以下是完整代码:
```c
#include <stdio.h>
#include <string.h>
#include <malloc.h>
#define ENDKEY -1
typedef struct node {
int key;
struct node *lchild, *rchild;
} BSTNode, *BSTree;
// 二叉排序树插入
void InsertBST(BSTree *bst, int key) {
BSTree s, p, q;
s = (BSTree)malloc(sizeof(BSTNode));
s->key = key;
s->lchild = s->rchild = NULL;
if (*bst == NULL) {
*bst = s;
} else {
p = *bst;
while (p != NULL) {
q = p;
if (key < p->key) {
p = p->lchild;
} else {
p = p->rchild;
}
}
if (key < q->key) {
q->lchild = s;
} else {
q->rchild = s;
}
}
}
// 创建二叉排序树
void CreatBST(BSTree *bst) {
int key;
*bst = NULL;
scanf("%d", &key);
while (key != ENDKEY) {
InsertBST(bst, key);
scanf("%d", &key);
}
}
// 中序遍历二叉树
void InOrder(BSTree bst) {
if (bst != NULL) {
InOrder(bst->lchild);
printf("%d ", bst->key);
InOrder(bst->rchild);
}
}
int main() {
BSTree bst;
CreatBST(&bst);
InOrder(bst);
return 0;
}
```
该代码使用二叉链表作为存储结构,实现了输入键值序列建立一棵二叉排序树,并且中序遍历输出二叉树。
#include <stdio.h> #include <string.h> #include <malloc.h> #define ENDKEY -1 typedef struct node { int key; struct node *lchild, *rchild; } BSTNode, *BSTree; // 二叉排序树插入 void InsertBST(BSTree *bst, int key) { BSTree s, p, q; s = (BSTree)malloc(sizeof(BSTNode)); s->key = key; s->lchild = s->rchild = NULL; if (*bst == NULL) { *bst = s; } else { p = *bst; while (p != NULL) { q = p; if (key < p->key) { p = p->lchild; } else { p = p->rchild; } } if (key < q->key) { q->lchild = s; } else { q->rchild = s; } } } // 创建二叉排序树 void CreatBST(BSTree *bst) { int key; *bst = NULL; scanf("%d", &key); while (key != ENDKEY) { InsertBST(bst, key); scanf("%d", &key); } } // 中序遍历二叉树 void InOrder(BSTree bst) { if (bst != NULL) { InOrder(bst->lchild); printf("%d ", bst->key); InOrder(bst->rchild); } } int main() { BSTree bst; CreatBST(&bst); InOrder(bst); return 0; }改正该代码的错误之处
该代码没有明确指出错误的具体位置,以下是我发现的可能的错误和对应的解决方法:
1. 在 `InsertBST` 函数中,没有处理当插入节点的键值与已有节点的键值相同时的情况。解决方法是,在遍历搜索树时,如果找到了一个键值与插入节点相同的节点,则不插入新节点,直接返回。
2. 在 `CreatBST` 函数中,使用了一个未定义的宏 `ENDKEY`。解决方法是,在程序开头定义宏 `ENDKEY`,例如 `#define ENDKEY -1`。
3. 在 `main` 函数中,没有检查动态内存分配是否成功。解决方法是,在调用 `malloc` 分配内存后,检查返回的指针是否为 NULL,如果是则说明分配失败,应该结束程序或进行相应的错误处理。
4. 在 `main` 函数中,没有释放动态分配的内存。解决方法是,在程序结束前,通过调用 `free` 函数释放动态分配的内存。
以下是修改后的代码:
```c
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#define ENDKEY -1
typedef struct node {
int key;
struct node *lchild, *rchild;
} BSTNode, *BSTree;
// 二叉排序树插入
void InsertBST(BSTree *bst, int key) {
BSTree s, p, q;
s = (BSTree)malloc(sizeof(BSTNode));
if (s == NULL) {
fprintf(stderr, "Error: failed to allocate memory\n");
exit(EXIT_FAILURE);
}
s->key = key;
s->lchild = s->rchild = NULL;
if (*bst == NULL) {
*bst = s;
} else {
p = *bst;
while (p != NULL) {
q = p;
if (key < p->key) {
p = p->lchild;
} else if (key > p->key) {
p = p->rchild;
} else {
free(s); // 释放已分配的节点内存
return; // 不插入新节点
}
}
if (key < q->key) {
q->lchild = s;
} else {
q->rchild = s;
}
}
}
// 创建二叉排序树
void CreatBST(BSTree *bst) {
int key;
*bst = NULL;
scanf("%d", &key);
while (key != ENDKEY) {
InsertBST(bst, key);
scanf("%d", &key);
}
}
// 中序遍历二叉树
void InOrder(BSTree bst) {
if (bst != NULL) {
InOrder(bst->lchild);
printf("%d ", bst->key);
InOrder(bst->rchild);
}
}
int main() {
BSTree bst;
CreatBST(&bst);
InOrder(bst);
free(bst); // 释放二叉树的内存
return 0;
}
```
阅读全文
相关推荐
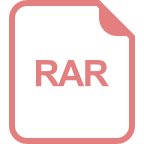
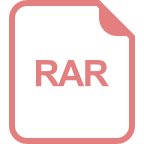
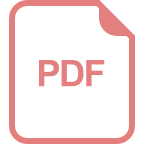


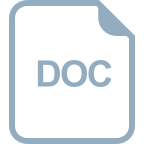










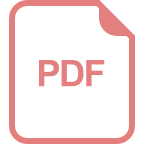
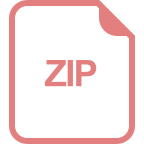