opencv c++ 直线拟合
时间: 2023-12-04 20:41:49 浏览: 168
以下是使用OpenCV C++实现RANSAC随机抽样一致性直线拟合的示例代码:
```cpp
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
// 生成随机点
Mat points(100, 1, CV_32FC2);
RNG& rng = theRNG();
for (int i = 0; i < points.rows; i++)
{
points.at<Vec2f>(i, 0)[0] = rng.uniform(0.f, (float)640);
points.at<Vec2f>(i, 0)[1] = rng.uniform(0.f, (float)480);
}
// 添加噪声
for (int i = 0; i < points.rows; i++)
{
if (i % 5 == 0)
{
points.at<Vec2f>(i, 0)[0] += rng.uniform(-50.f, 50.f);
points.at<Vec2f>(i, 0)[1] += rng.uniform(-50.f, 50.f);
}
}
// RANSAC 直线拟合
Vec4f line;
fitLine(points, line, DIST_L2, 0, 0.01, 0.01);
// 绘制拟合直线和随机点
Mat img(480, 640, CV_8UC3, Scalar::all(0));
for (int i = 0; i < points.rows; i++)
{
circle(img, Point(points.at<Vec2f>(i, 0)[0], points.at<Vec2f>(i, 0)[1]), 2, Scalar(0, 255, 0), -1, LINE_AA);
}
float k = line[1] / line[0];
float b = line[3] - k * line[2];
Point pt1(0, b);
Point pt2(img.cols, k * img.cols + b);
line(img, pt1, pt2, Scalar(0, 0, 255), 2, LINE_AA);
// 显示结果
imshow("line fitting", img);
waitKey(0);
return 0;
}
```
阅读全文
相关推荐
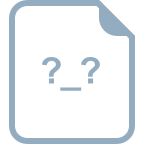


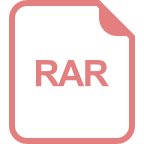
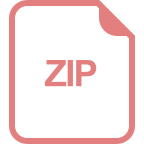
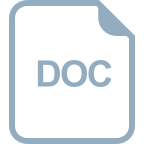
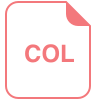









