Student(string id, int score) { this->id = id; this->score = score; }
时间: 2024-06-01 14:11:39 浏览: 65
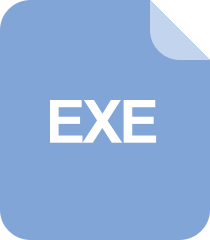
学生数据输入
This code defines a constructor function for a class called "Student". The constructor takes two parameters, a string called "id" and an integer called "score". Within the constructor, the "id" and "score" parameters are assigned to the corresponding member variables of the class using the "this" pointer. This allows the member variables to be accessed and modified within the scope of the constructor function.
阅读全文
相关推荐
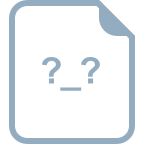















