编写一个程序,使用邻接表法定义图并实现图的深度优先遍历算法,并在主函数中输出下图从顶点A开始的一个深度优先遍历序列。 C++
时间: 2024-12-13 12:24:50 浏览: 23
在C++中,我们可以使用邻接表(Adjacency List)数据结构来定义无向图,然后实现深度优先搜索(Depth-First Search, DFS)。这里是一个简单的示例,首先创建邻接表表示图,然后执行DFS遍历:
```cpp
#include <iostream>
#include <vector>
#include <stack>
// 邻接表节点
struct Node {
int vertex;
std::vector<int> neighbors; // 存储相邻的顶点
};
// 创建图
std::vector<Node> createGraph() {
std::vector<Node> graph = { // 从顶点A开始的图
{ 'A', { 'B', 'C' } },
{ 'B', { 'D' } },
{ 'C', {} },
{ 'D', {} }
};
return graph;
}
// 深度优先遍历
void dfs(Node* node, char start, std::string& result) {
if (node->vertex == start) {
result += start;
std::stack<Node*> stack;
stack.push(node);
while (!stack.empty()) {
node = stack.top();
stack.pop();
for (int neighbor : node->neighbors) {
if (result.find(neighbor) == std::string::npos) {
result += " -> " + neighbor;
stack.push(graph[neighbor - 'A']);
}
}
}
}
}
// 主函数输出深度优先遍历序列
int main() {
std::vector<Node> graph = createGraph();
std::string dfs_sequence = "";
dfs(&graph[0], 'A', dfs_sequence);
std::cout << "深度优先遍历序列: " << dfs_sequence << std::endl;
return 0;
}
```
在这个例子中,我们首先创建了一个表示给定图的邻接表。`dfs`函数接收当前节点、起点以及结果字符串,它会访问所有从起点A可达的顶点并添加到序列中。在`main`函数里,我们将顶点A作为起点,执行深度优先遍历并将结果打印出来。
阅读全文
相关推荐
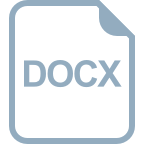
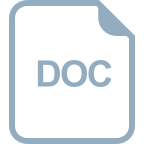
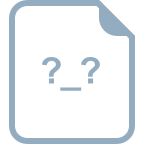















