将python代码 if max(np.max(E - E_old) / np.max(X), np.max(W - W_old) / np.max(X), np.max(Z - Z_old) / np.max(X), np.max(P - P_old) / np.max(X), np.max(D - D_old) / np.max(X)) < 1e-6 or max(np.max(X - D - E) / np.max(X), np.max(X - D - E) / np.max(X)) < 1e-7: break else: t = t + 1转为matlab
时间: 2024-01-11 13:05:29 浏览: 101
以下是将该Python代码转换为MATLAB代码的结果:
```
while true
if max([max(max((E - E_old) ./ X)), max(max((W - W_old) ./ X)), ...
max(max((Z - Z_old) ./ X)), max(max((P - P_old) ./ X)), ...
max(max((D - D_old) ./ X))]) < 1e-6 || ...
max([max(max((X - D - E) ./ X)), max(max((X - D - E) ./ X))]) < 1e-7
break;
else
t = t + 1;
end
end
```
注意,MATLAB中的矩阵除法运算符为“./”,而不是Python中的“/”。
相关问题
上述代码报错:Using TensorFlow backend. 2023-06-02 17:07:57.266774: W tensorflow/stream_executor/platform/default/dso_loader.cc:64] Could not load dynamic library 'cudart64_110.dll'; dlerror: cudart64_110.dll not found 2023-06-02 17:07:57.267572: I tensorflow/stream_executor/cuda/cudart_stub.cc:29] Ignore above cudart dlerror if you do not have a GPU set up on your machine. Loading data... Traceback (most recent call last): File "C:/Users/85448/PycharmProjects/LightGBM_text/deep2.py", line 12, in <module> (x_train, y_train), (x_test, y_test) = imdb.load_data(num_words=max_features) File "C:\Users\85448\anaconda3\envs\tensorflow37\lib\site-packages\keras\datasets\imdb.py", line 54, in load_data x_train, labels_train = f['x_train'], f['y_train'] File "C:\Users\85448\anaconda3\envs\tensorflow37\lib\site-packages\numpy\lib\npyio.py", line 256, in __getitem__ pickle_kwargs=self.pickle_kwargs) File "C:\Users\85448\anaconda3\envs\tensorflow37\lib\site-packages\numpy\lib\format.py", line 743, in read_array raise ValueError("Object arrays cannot be loaded when " ValueError: Object arrays cannot be loaded when allow_pickle=False
这个错误看起来像是 numpy 版本不兼容导致的问题。您可以试着升级 numpy 版本,或者添加一行代码来解决这个问题:
```
import numpy as np
np_load_old = np.load
np.load = lambda *a,**k: np_load_old(*a, allow_pickle=True, **k)
```
然后在加载IMDB数据集之前添加以上代码即可,例如:
```python
import numpy as np
np_load_old = np.load
np.load = lambda *a,**k: np_load_old(*a, allow_pickle=True, **k)
from keras.datasets import imdb
from keras.preprocessing import sequence
from keras.models import Sequential
from keras.layers import Dense, Embedding, LSTM
# 加载数据集
max_features = 20000
maxlen = 80
batch_size = 32
print('Loading data...')
(x_train, y_train), (x_test, y_test) = imdb.load_data(num_words=max_features)
print(len(x_train), 'train sequences')
print(len(x_test), 'test sequences')
# 填充序列,使其长度一致
print('Pad sequences (samples x time)')
x_train = sequence.pad_sequences(x_train, maxlen=maxlen)
x_test = sequence.pad_sequences(x_test, maxlen=maxlen)
print('x_train shape:', x_train.shape)
print('x_test shape:', x_test.shape)
# 构建模型
model = Sequential()
model.add(Embedding(max_features, 128, input_length=maxlen))
model.add(LSTM(128, dropout=0.2, recurrent_dropout=0.2))
model.add(Dense(1, activation='sigmoid'))
model.compile(loss='binary_crossentropy',
optimizer='adam',
metrics=['accuracy'])
# 训练模型
print('Train...')
model.fit(x_train, y_train,
batch_size=batch_size,
epochs=15,
validation_data=(x_test, y_test))
# 评估模型
score, acc = model.evaluate(x_test, y_test,
batch_size=batch_size)
print('Test score:', score)
print('Test accuracy:', acc)
np.load = np_load_old # 恢复原来的 numpy.load 方法
```
如果还有问题,请随时告诉我。
按上述修改后报错D:\mycode2\PPO-PyTorch\PPO.py:52: VisibleDeprecationWarning: Creating an ndarray from ragged nested sequences (which is a list-or-tuple of lists-or-tuples-or ndarrays with different lengths or shapes) is deprecated. If you meant to do this, you must specify 'dtype=object' when creating the ndarray. state = np.array([np.array(sublist) for sublist in state]) Traceback (most recent call last): File "D:\mycode2\PPO-PyTorch\PPO.py", line 210, in <module> main() File "D:\mycode2\PPO-PyTorch\PPO.py", line 173, in main action = ppo.policy_old.act(state, memory) File "D:\mycode2\PPO-PyTorch\PPO.py", line 53, in act state = torch.from_numpy(state).float().to(device) TypeError: can't convert np.ndarray of type numpy.object_. The only supported types are: float64, float32, float16, complex64, complex128, int64, int32, int16, int8, uint8, and bool.
非常抱歉,我之前提供的解决方案似乎没有解决问题。
根据报错信息,你的 `state` 数组中仍然包含不规则的嵌套序列,导致无法将其转换为 NumPy 数组。为了解决这个问题,你可以尝试使用其他方法来处理 `state` 数组。
一种方法是使用 `np.asarray()` 函数来创建一个包含 `state` 内容的 NumPy 数组,并指定 `dtype=object` 参数以处理不规则的嵌套序列。修改代码如下:
```python
state = np.asarray(state, dtype=object)
state = torch.from_numpy(state).float().to(device)
```
另一种方法是先检查 `state` 数组的形状,并确保所有子数组的长度相等。然后使用 `np.array()` 函数将其转换为 NumPy 数组。例如,假设 `state` 是一个嵌套列表,你可以使用以下代码进行转换:
```python
max_length = max(len(sublist) for sublist in state)
state = np.array([sublist + [0] * (max_length - len(sublist)) for sublist in state])
state = torch.from_numpy(state).float().to(device)
```
这样做将会通过在每个子列表末尾添加零填充,使得所有子列表具有相同的长度,并将其转换为规则的二维数组。
请尝试按照上述方式修改代码,并重新运行程序。如果问题仍然存在,请提供更多关于 `state` 数组的信息,以便我能够更好地帮助你解决问题。谢谢!
阅读全文
相关推荐
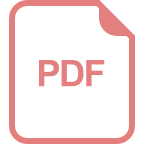
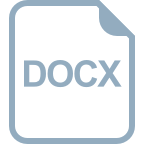
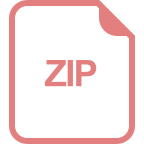













