如何算a^ mod 1000000007逆元(int范围以内)
时间: 2024-09-28 08:10:05 浏览: 33
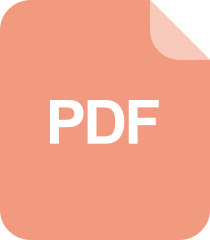
有限域逆元算法的实现

要计算一个数 \( a \) 对于模数 \( 1000000007 \) 的逆元,你需要找到一个整数 \( b \),使得 \( a \times b \equiv 1 \pmod{1000000007} \)。这是一个扩展欧几里得算法或更常见的是中国剩余定理的问题,但在这里我们将使用更基础的方法,特别是对于小范围的模数。
首先,确保 \( a \) 和 \( 1000000007 \) 互质,因为如果它们有公共因子,\( a \) 就没有逆元。然后,你可以用扩展欧几里得算法来找到 \( b \),该算法可以在线性时间内完成。
以下是一个简单的 C++ 实现:
```cpp
#include <iostream>
using namespace std;
// 计算最大公约数
int gcd(int a, int b) {
if (b == 0)
return a;
return gcd(b, a % b);
}
// 计算两个数的最大公约数并返回a除以最大公约数的商
pair<int, int> extendedEuclidean(int a, int b) {
if (b == 0) {
return {a, 1};
} else {
auto result = extendedEuclidean(b, a % b);
int x = result.second;
int y = result.first - a / b * x;
return {y, x};
}
}
// 计算a的逆元mod 1000000007
int modularInverse(int a) {
// 检查a是否为0,如果是则没有逆元
if (a == 0) {
throw runtime_error("Modular inverse does not exist for zero.");
}
int mod = 1000000007;
pair<int, int> res = extendedEuclidean(a, mod);
return (res.first + mod) % mod; // 因为x可能负,加mod确保结果在[0, mod-1]内
}
int main() {
try {
int a;
cout << "Enter the number: ";
cin >> a;
cout << "The modular inverse of " << a << " mod 1000000007 is: " << modularInverse(a) << endl;
} catch (const runtime_error& e) {
cerr << "Error: " << e.what() << endl;
}
return 0;
}
```
运行这个程序时,用户可以输入他们想要找逆元的数 \( a \),程序将输出相应的逆元。
阅读全文
相关推荐
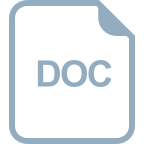
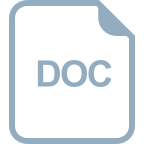















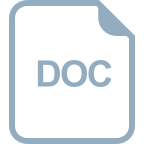