在Linux系统下写一个C语言程序,用户可以自己输入一段格式为:$GNRMC,<1>,<2>,<3>,<4>,<5>,<6>,<7>,<8>,<9>,,,<11>,<12>*hh<CR><LF>的数据,程序通过数逗号的方式解析出数据中的日期和年月日信息,<1>是UTC时间,hhmmss.sss格式,<9>是UTC日期,DDMMYY格式,读取到以后将日期和时间转换为北京时间和日期并输入。
时间: 2023-08-17 09:38:04 浏览: 90
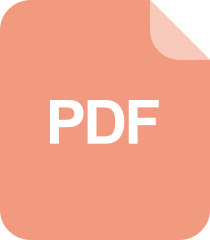
如何在Linux上写C程序
好的,以下是一个可以实现这个功能的C语言程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#define MAX_INPUT_LENGTH 200
// 函数声明
int parse_utc_time(char* time_str, struct tm* utc_time);
int parse_utc_date(char* date_str, struct tm* utc_time);
void print_beijing_time(struct tm* utc_time);
int main() {
// 读取用户输入的数据
char input[MAX_INPUT_LENGTH];
printf("请输入格式为 $GNRMC,<1>,<2>,<3>,<4>,<5>,<6>,<7>,<8>,<9>,,,<11>,<12>*hh<CR><LF> 的数据:\n");
fgets(input, MAX_INPUT_LENGTH, stdin);
// 解析数据中的日期和时间
char* tokens[13];
char* token = strtok(input, ",");
int i = 0;
while (token != NULL && i < 13) {
tokens[i++] = token;
token = strtok(NULL, ",");
}
if (i < 10) {
printf("输入数据不完整!\n");
return 1;
}
struct tm utc_time;
memset(&utc_time, 0, sizeof(struct tm));
// 解析时间和日期
if (!parse_utc_time(tokens[0], &utc_time)) {
printf("时间格式错误!\n");
return 1;
}
if (!parse_utc_date(tokens[8], &utc_time)) {
printf("日期格式错误!\n");
return 1;
}
// 转换为北京时间
utc_time.tm_hour += 8;
if (utc_time.tm_hour >= 24) {
utc_time.tm_hour -= 24;
utc_time.tm_mday += 1;
}
// 输出北京时间
print_beijing_time(&utc_time);
return 0;
}
// 解析UTC时间字符串,返回0表示解析失败,返回1表示解析成功
int parse_utc_time(char* time_str, struct tm* utc_time) {
if (strlen(time_str) != 9) {
return 0;
}
char* endptr;
int hour = strtol(time_str, &endptr, 10);
if (endptr == time_str) {
return 0;
}
if (*endptr != '\0' && *(endptr + 1) != '.') {
return 0;
}
int minute = strtol(endptr + 1, &endptr, 10);
if (endptr == time_str + 6) {
return 0;
}
if (*endptr != '\0' && *(endptr + 1) != '.') {
return 0;
}
int second = strtol(endptr + 1, &endptr, 10);
if (endptr == time_str + 9) {
return 0;
}
utc_time->tm_hour = hour;
utc_time->tm_min = minute;
utc_time->tm_sec = second;
return 1;
}
// 解析UTC日期字符串,返回0表示解析失败,返回1表示解析成功
int parse_utc_date(char* date_str, struct tm* utc_time) {
if (strlen(date_str) != 6) {
return 0;
}
char* endptr;
int day = strtol(date_str, &endptr, 10);
if (endptr == date_str) {
return 0;
}
if (*endptr != '\0' && *(endptr + 1) != '\0') {
return 0;
}
int month = strtol(endptr + 1, &endptr, 10);
if (endptr == date_str + 4) {
return 0;
}
if (*endptr != '\0' && *(endptr + 1) != '\0') {
return 0;
}
int year = strtol(endptr + 1, &endptr, 10);
if (endptr == date_str + 6) {
return 0;
}
if (year >= 80 && year <= 99) {
year += 1900;
} else {
year += 2000;
}
utc_time->tm_mday = day;
utc_time->tm_mon = month - 1;
utc_time->tm_year = year - 1900;
return 1;
}
// 输出北京时间
void print_beijing_time(struct tm* utc_time) {
time_t utc_timestamp = mktime(utc_time);
struct tm* beijing_time = localtime(&utc_timestamp);
char time_str[20];
strftime(time_str, sizeof(time_str), "%Y-%m-%d %H:%M:%S", beijing_time);
printf("北京时间:%s\n", time_str);
}
```
这个程序先读取用户输入的数据,然后通过逗号分隔符解析出其中的时间和日期信息。接着,它会调用 `parse_utc_time` 和 `parse_utc_date` 函数来解析时间和日期字符串,并将解析结果存储到一个 `tm` 结构体中。最后,程序会将 UTC 时间转换为北京时间,并输出结果。
注意,在这个程序中,我们使用了标准库函数 `strtol` 来解析整数。`strtol` 函数可以将一个字符串解析为一个整数,并返回解析结果。如果解析失败,它会返回 `0`。另外,我们还使用了标准库函数 `mktime` 和 `localtime` 来将时间戳转换为 `tm` 结构体,并将 UTC 时间转换为北京时间。
阅读全文
相关推荐
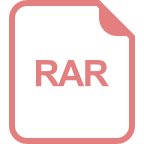
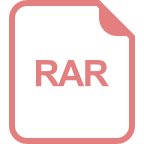
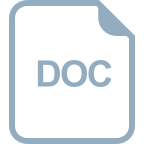
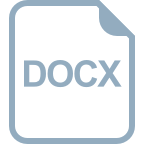
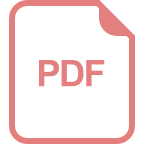
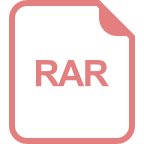
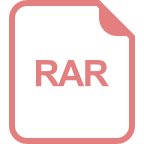
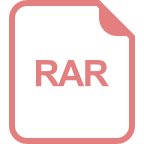
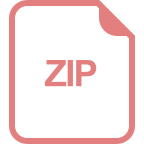
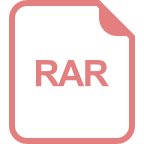
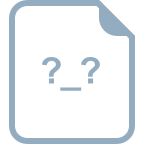
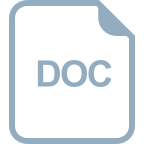
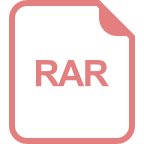
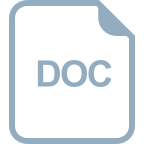
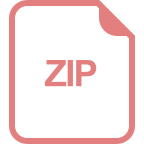
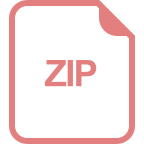