无人机路径规划A*算法代码
时间: 2023-06-30 11:14:09 浏览: 58
A*算法是一种启发式搜索算法,可以用于无人机路径规划。以下是一个简单的A*算法的Python代码实现:
```
# 定义A*算法函数
def A_star(start, end, h_func, move_func):
# 初始化起点和终点的节点
start_node = Node(start, None)
start_node.g = start_node.h = start_node.f = 0
end_node = Node(end, None)
end_node.g = end_node.h = end_node.f = 0
# 定义开放和关闭节点列表
open_list = []
closed_list = []
# 将起点加入开放列表
open_list.append(start_node)
# 循环直到找到终点或开放列表为空
while len(open_list) > 0:
# 从开放列表中找到f值最小的节点
current_node = open_list[0]
current_index = 0
for index, item in enumerate(open_list):
if item.f < current_node.f:
current_node = item
current_index = index
# 将当前节点从开放列表中删除,并加入关闭列表
open_list.pop(current_index)
closed_list.append(current_node)
# 如果找到了终点,则返回路径
if current_node == end_node:
path = []
current = current_node
while current is not None:
path.append(current.position)
current = current.parent
return path[::-1]
# 获取当前节点周围的可行节点
children = []
for new_position in move_func(current_node.position):
# 创建新的节点
node = Node(new_position, current_node)
# 检查新节点是否在关闭列表中
if node in closed_list:
continue
# 计算新节点的g、h和f值
node.g = current_node.g + 1
node.h = h_func(node.position, end_node.position)
node.f = node.g + node.h
# 检查新节点是否已经在开放列表中,并更新g值
for open_node in open_list:
if node == open_node and node.g > open_node.g:
continue
# 将新节点加入开放列表
open_list.append(node)
# 如果开放列表为空,则表示没有找到路径
return None
# 定义节点类
class Node:
def __init__(self, position, parent):
self.position = position
self.parent = parent
self.g = 0
self.h = 0
self.f = 0
def __eq__(self, other):
return self.position == other.position
# 定义移动函数
def move_func(position):
moves = []
x, y = position
for dx, dy in [(0, 1), (0, -1), (1, 0), (-1, 0)]:
new_x, new_y = x + dx, y + dy
if 0 <= new_x < 10 and 0 <= new_y < 10:
moves.append((new_x, new_y))
return moves
# 定义启发函数(曼哈顿距离)
def h_func(position, end_position):
return abs(position[0] - end_position[0]) + abs(position[1] - end_position[1])
```
在上面的代码中,我们定义了一个A_star函数,它接受起点、终点、启发函数和移动函数作为参数,并返回从起点到终点的最短路径。我们还定义了一个Node类来表示节点。在A_star函数中,我们使用了开放列表和关闭列表来实现A*算法。我们还定义了一个移动函数,它返回一个节点周围的可行节点。我们使用曼哈顿距离作为启发函数来估计每个节点到终点的距离。
相关推荐
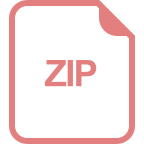
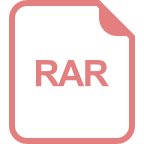














