hevc流中解析nal header,slice类型 代码实现
时间: 2023-07-15 08:11:59 浏览: 165
下面是一个简单的 C++ 代码示例,用于解析 HEVC 流中的 NAL 头和切片类型:
```cpp
#include <iostream>
#include <fstream>
#include <cstring>
using namespace std;
// NAL 头结构体
struct NALHeader {
int forbidden_zero_bit;
int nal_unit_type;
int nuh_layer_id;
int nuh_temporal_id_plus1;
};
// 切片类型枚举
enum SliceType {
B_SLICE = 0,
P_SLICE = 1,
I_SLICE = 2,
IDR_SLICE = 3,
SP_SLICE = 4,
SI_SLICE = 5,
P_SLICE_BLA = 6,
P_SLICE_BLA_RET = 7,
P_SLICE_BLA_N_LP = 8,
P_SLICE_IDR = 9,
B_SLICE_BLA = 10,
B_SLICE_BLA_RET = 11,
B_SLICE_BLA_N_LP = 12,
IDR_SLICE_BLA = 13,
IDR_SLICE_BLA_RET = 14,
IDR_SLICE_BLA_N_LP = 15,
};
int main() {
ifstream ifs("sample.hevc", ios::binary); // 打开 HEVC 流文件
if (!ifs) {
cerr << "Error opening file." << endl;
return -1;
}
char buffer[1024];
int nal_unit_type;
SliceType slice_type;
while (ifs.read(buffer, sizeof(buffer))) {
for (int i = 0; i < ifs.gcount(); ) {
// 查找 NAL 头起始位置
while (i < ifs.gcount() - 3 && !(buffer[i] == 0x00 && buffer[i+1] == 0x00 && buffer[i+2] == 0x01)) {
i++;
}
if (i >= ifs.gcount() - 3) {
break;
}
// 解析 NAL 头
NALHeader nal_header;
nal_header.forbidden_zero_bit = (buffer[i+3] >> 7) & 0x01;
nal_header.nal_unit_type = (buffer[i+3] >> 1) & 0x3f;
nal_header.nuh_layer_id = (buffer[i+4] >> 1) & 0x3f;
nal_header.nuh_temporal_id_plus1 = buffer[i+5] & 0x07;
// 获取切片类型
if (nal_header.nal_unit_type >= 16 && nal_header.nal_unit_type <= 21) {
slice_type = static_cast<SliceType>(nal_header.nal_unit_type - 16);
} else if (nal_header.nal_unit_type >= 32 && nal_header.nal_unit_type <= 34) {
slice_type = static_cast<SliceType>(nal_header.nal_unit_type - 24);
} else {
slice_type = static_cast<SliceType>(-1);
}
// 输出 NAL 头和切片类型
cout << "NAL Header: forbidden_zero_bit=" << nal_header.forbidden_zero_bit
<< ", nal_unit_type=" << nal_header.nal_unit_type
<< ", nuh_layer_id=" << nal_header.nuh_layer_id
<< ", nuh_temporal_id_plus1=" << nal_header.nuh_temporal_id_plus1 << endl;
cout << "Slice Type: " << slice_type << endl;
// 移动指针到下一个 NAL 头
i += 3;
}
}
ifs.close();
return 0;
}
```
代码中使用了 ifstream 类来打开 HEVC 流文件,然后读取数据并解析 NAL 头和切片类型。NAL 头包含了 forbidden_zero_bit、nal_unit_type、nuh_layer_id 和 nuh_temporal_id_plus1 等字段。切片类型用枚举类型 SliceType 表示。在代码中,我们使用了查找 0x00 0x00 0x01 的方式来定位 NAL 头的起始位置。这种方式并不是 HEVC 标准规定的方法,在实际应用中需要根据标准规范来实现。
阅读全文
相关推荐
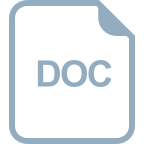
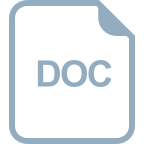
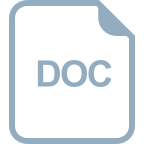








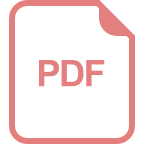
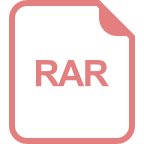
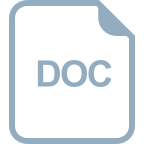




