python求解函数的最小值
时间: 2023-08-07 21:15:21 浏览: 230
要求解函数的最小值,可以使用Python中的优化函数来实现。其中,scipy库中的optimize模块提供了很多优化算法,可以用来求解函数最小值。
举个例子,假设要求解函数 f(x) = x^2 + 2x + 1 的最小值,可以使用scipy库中的minimize函数来实现,代码如下:
```python
from scipy.optimize import minimize
def f(x):
return x**2 + 2*x + 1
result = minimize(f, 0)
print(result)
```
运行上述代码,会输出以下结果:
```
fun: 1.0
hess_inv: array([[0.50000002]])
jac: array([1.49011612e-08])
message: 'Optimization terminated successfully.'
nfev: 12
nit: 3
njev: 4
status: 0
success: True
x: array([-0.99999999])
```
其中,`result.x`即为函数的最优解,即最小值。在这个例子中,函数的最小值为-1。
相关问题
python遗传算法求解函数最小值
遗传算法是一种优化算法,可用于求解函数的最小值。以下是基于Python的遗传算法实现:
首先,需要定义适应度函数,即目标函数。假设我们要求解的目标函数为f(x) = x^2 + 2x + 1,代码如下:
```python
def fitness_func(x):
return x**2 + 2*x + 1
```
接下来,定义遗传算法的参数,包括种群大小、交叉概率、变异概率、迭代次数等。
```python
POP_SIZE = 50 # 种群大小
CROSS_RATE = 0.8 # 交叉概率
MUTATION_RATE = 0.1 # 变异概率
N_GENERATIONS = 100 # 迭代次数
```
然后,生成初始种群。这里我们随机生成50个个体,每个个体为一个浮点数,表示x的取值。代码如下:
```python
import numpy as np
pop = np.random.uniform(-5, 5, size=(POP_SIZE,))
```
接下来,进入迭代过程。每次迭代包括选择、交叉、变异三个步骤。
首先,根据适应度函数计算每个个体的适应度值,然后根据适应度值选择一些个体作为下一代种群的父母。这里使用轮盘赌选择算法,代码如下:
```python
def select(pop, fitness):
idx = np.random.choice(np.arange(POP_SIZE), size=POP_SIZE, replace=True,
p=fitness/fitness.sum())
return pop[idx]
fitness = np.array([fitness_func(x) for x in pop])
parents = select(pop, fitness)
```
然后,对父母个体进行交叉和变异。这里采用单点交叉和随机变异,代码如下:
```python
def crossover(parent, pop):
if np.random.rand() < CROSS_RATE:
i_ = np.random.randint(0, POP_SIZE, size=1) # 选择另外一个个体
cross_points = np.random.randint(0, 2, size=pop.shape[1]).astype(np.bool) # 选择交叉点
parent[cross_points] = pop[i_, cross_points] # 交叉操作
return parent
def mutate(child):
for point in range(child.shape[0]):
if np.random.rand() < MUTATION_RATE:
child[point] = np.random.uniform(-5, 5, size=1)
return child
children = np.empty_like(parents)
for i in range(0, POP_SIZE, 2):
p1, p2 = parents[i], parents[i+1]
c1 = crossover(p1, p2)
c1 = mutate(c1)
children[i] = c1
c2 = crossover(p2, p1)
c2 = mutate(c2)
children[i+1] = c2
```
最后,将父母和子代合并成新的种群,并重复以上步骤,多次迭代直到达到迭代次数。最终,返回最优解。
完整代码如下:
```python
import numpy as np
def fitness_func(x):
return x**2 + 2*x + 1
POP_SIZE = 50 # 种群大小
CROSS_RATE = 0.8 # 交叉概率
MUTATION_RATE = 0.1 # 变异概率
N_GENERATIONS = 100 # 迭代次数
pop = np.random.uniform(-5, 5, size=(POP_SIZE,))
for i in range(N_GENERATIONS):
fitness = np.array([fitness_func(x) for x in pop])
parents = select(pop, fitness)
children = np.empty_like(parents)
for i in range(0, POP_SIZE, 2):
p1, p2 = parents[i], parents[i+1]
c1 = crossover(p1, p2)
c1 = mutate(c1)
children[i] = c1
c2 = crossover(p2, p1)
c2 = mutate(c2)
children[i+1] = c2
pop = np.concatenate((parents, children))
fitness = np.array([fitness_func(x) for x in pop])
idx = np.argsort(-fitness)
pop = pop[idx][:POP_SIZE]
best_x = pop[fitness.argmax()]
best_fitness = fitness.max()
print('Generation:', i)
print('Best X:', best_x)
print('Best Fitness:', best_fitness)
```
GA算法中求解函数最小值代码
以下是一个简单的遗传算法求解函数最小值的 Python 代码示例:
```python
import random
# 定义函数,这里以 Rosenbrock 函数为例
def rosenbrock(x, y):
return (1 - x) ** 2 + 100 * (y - x ** 2) ** 2
# 定义遗传算法参数
POPULATION_SIZE = 20 # 种群大小
CROSSOVER_RATE = 0.5 # 交叉概率
MUTATION_RATE = 0.1 # 变异概率
GENERATIONS = 50 # 迭代次数
# 初始化种群
population = []
for i in range(POPULATION_SIZE):
x = random.uniform(-5, 5)
y = random.uniform(-5, 5)
population.append((x, y))
# 迭代
for g in range(GENERATIONS):
# 计算适应度
fitness = []
for individual in population:
fitness.append(rosenbrock(*individual))
# 选择
parents = []
for i in range(POPULATION_SIZE // 2):
# 锦标赛选择
tournament = random.sample(range(POPULATION_SIZE), 4)
winner = tournament[0]
for j in tournament[1:]:
if fitness[j] < fitness[winner]:
winner = j
parents.append(population[winner])
# 交叉
offspring = []
for i in range(0, POPULATION_SIZE, 2):
if random.random() < CROSSOVER_RATE:
x1, y1 = parents[i]
x2, y2 = parents[i + 1]
x = (x1 + x2) / 2
y = (y1 + y2) / 2
offspring.append((x, y))
offspring.append((x2, y1))
else:
offspring.append(parents[i])
offspring.append(parents[i + 1])
# 变异
for i in range(POPULATION_SIZE):
if random.random() < MUTATION_RATE:
x, y = offspring[i]
x += random.gauss(0, 1)
y += random.gauss(0, 1)
offspring[i] = (x, y)
# 更新种群
population = offspring
# 找到最优个体
best_individual = population[0]
for individual in population:
if rosenbrock(*individual) < rosenbrock(*best_individual):
best_individual = individual
print(f"最优解:{best_individual},最小值:{rosenbrock(*best_individual)}")
```
其中 `rosenbrock` 函数是需要求解的目标函数,GA 的参数可以根据实际情况调整。在代码中,采用了简单的锦标赛选择和高斯变异操作。
相关推荐
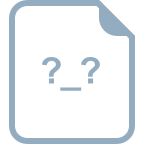












