用C语言编写链队列其他操作的实现和调用 QueueEmpty(Q)条件:队列Q已存在,结果:若Q为空队列,则 返回TRUE,否则返回FALSE QueueLength(Q)返回Q的元素个数,即队列的长度GetHead(Q,&e)用e返回Q的队头元素
时间: 2024-10-10 17:08:12 浏览: 15
在C语言中,我们可以使用结构体和指针来实现链队列(LinkedList Queue)。首先,定义一个节点结构体:
```c
typedef struct Node {
void* data; // 存储数据
struct Node* next; // 指向下一个节点
} Node;
```
然后,创建链队列的结构,并包含一些基本的操作函数:
```c
typedef struct Queue {
Node* front; // 队头指针
Node* rear; // 队尾指针
int length; // 队列长度
} Queue;
// 初始化空队列
Queue* initQueue() {
Queue* q = (Queue*)malloc(sizeof(Queue));
q->front = NULL;
q->rear = NULL;
q->length = 0;
return q;
}
// 判断队列是否为空
int isQueueEmpty(Queue* q) {
return q->front == NULL;
}
// 返回队列长度
int queueLength(Queue* q) {
return q->length;
}
// 获取队头元素并移动队头
void* getHead(Queue* q, void** e) {
if (isQueueEmpty(q)) {
*e = NULL;
return NULL;
}
*e = q->front->data;
Node* temp = q->front;
q->front = q->front->next;
if (q->front == NULL)
q->rear = NULL;
else
q->rear->next = NULL;
q->length--;
return *e;
}
// 入队操作(在队尾添加)
void enqueue(Queue* q, void* data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
if (isQueueEmpty(q))
q->front = q->rear = newNode;
else {
q->rear->next = newNode;
q->rear = newNode;
}
q->length++;
}
```
阅读全文
相关推荐
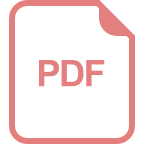
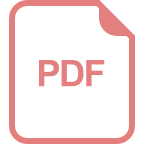
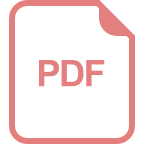















