2. 构建顺序表类Sqlist(参考课件“顺序表”代码)。执行以下操作: a) 将序列A={1,2,3,4,5,6,7,8}存入顺序表中:用java
时间: 2024-10-22 07:18:24 浏览: 28
为了构建一个简单的顺序表(Sequence List),你可以按照以下步骤在Java中创建一个`Sqlist`类,并将序列A={1, 2, 3, 4, 5, 6, 7, 8}存入其中:
首先,你需要定义一个`Node`类作为顺序表的节点,包含数据域和指向下一个节点的指针:
```java
class Node {
int data;
Node next;
// 构造函数和getter/setter省略
}
```
然后,定义`Sqlist`类,包括插入元素、查找等基本操作:
```java
public class Sqlist {
private Node head; // 链表头结点
private int size; // 序列长度
// 初始化一个空的顺序表
public Sqlist() {
head = null;
size = 0;
}
// 插入元素到列表尾部
public void add(int value) {
Node newNode = new Node();
newNode.data = value;
newNode.next = null;
if (head == null) {
head = newNode;
} else {
Node current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
size++;
}
// 将序列A添加到顺序表中
public void insertArray(int[] array) {
for (int num : array) {
add(num);
}
}
// 输出当前顺序表的内容
public void printList() {
Node current = head;
while (current != null) {
System.out.print(current.data + " -> ");
current = current.next;
}
System.out.println("null");
}
// 其他方法(如查找、删除等)可以根据需求添加
// 示例:将序列A存入顺序表
public static void main(String[] args) {
Sqlist list = new Sqlist();
int[] sequenceA = {1, 2, 3, 4, 5, 6, 7, 8};
list.insertArray(sequenceA);
list.printList();
}
}
```
在这个例子中,`main`方法创建了一个新的`Sqlist`对象,并通过`insertArray`方法将数组A的内容逐个插入。最后,`printList`方法用于检查顺序表是否正确存储了序列A的数据。
阅读全文
相关推荐
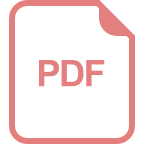
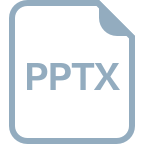
















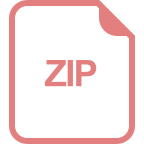
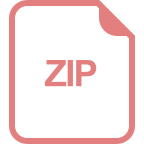