为什么使用pthread_cond_wait前需要调用pthread_mutex_lock?
时间: 2024-04-12 14:23:01 浏览: 109
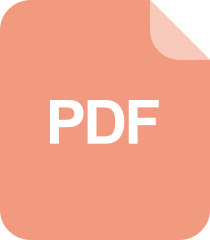
pthread_cond_wait() 用法深入分析
在使用pthread_cond_wait函数之前,需要先调用pthread_mutex_lock函数来获取互斥锁。这是因为pthread_cond_wait函数的工作机制需要依赖互斥锁来保证线程的同步和互斥。
pthread_cond_wait函数用于线程的条件等待,它会使当前线程进入等待状态,直到满足某个条件才会被唤醒。在调用pthread_cond_wait函数之前,需要先获取互斥锁,以确保在等待期间其他线程不会修改共享资源。
具体的流程如下:
1. 线程A调用pthread_mutex_lock函数获取互斥锁,成功获取后继续执行。
2. 线程A调用pthread_cond_wait函数,此时会自动释放之前获取的互斥锁,并进入等待状态。
3. 在其他线程中,当满足某个条件时,调用pthread_cond_signal或pthread_cond_broadcast函数来唤醒等待的线程A。
4. 线程A被唤醒后,会再次尝试获取之前释放的互斥锁,成功获取后继续执行。
通过使用互斥锁和条件变量的组合,可以实现线程之间的同步和通信。互斥锁用于保护共享资源的访问,而条件变量用于线程之间的等待和唤醒操作。
阅读全文
相关推荐
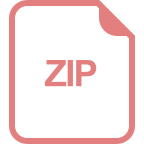
















